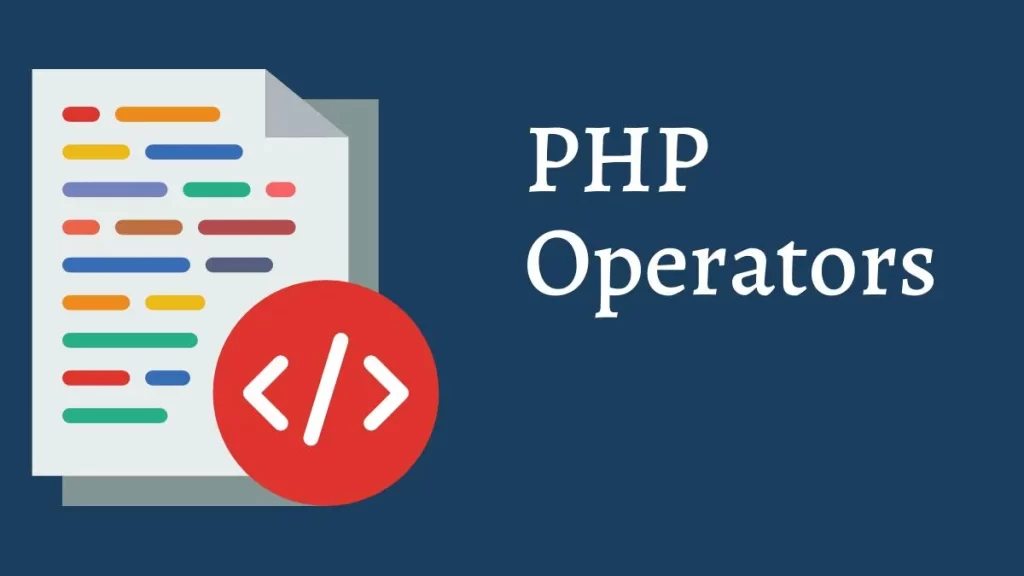
In PHP, Operators are special symbols that are used to perform operations on variables and values.
PHP has various types of operators-
- Arithmetic operator
- Comparison operator
- Logical operator
- Assignment operator
- Increment and Decrement operator
- Bitwise operator
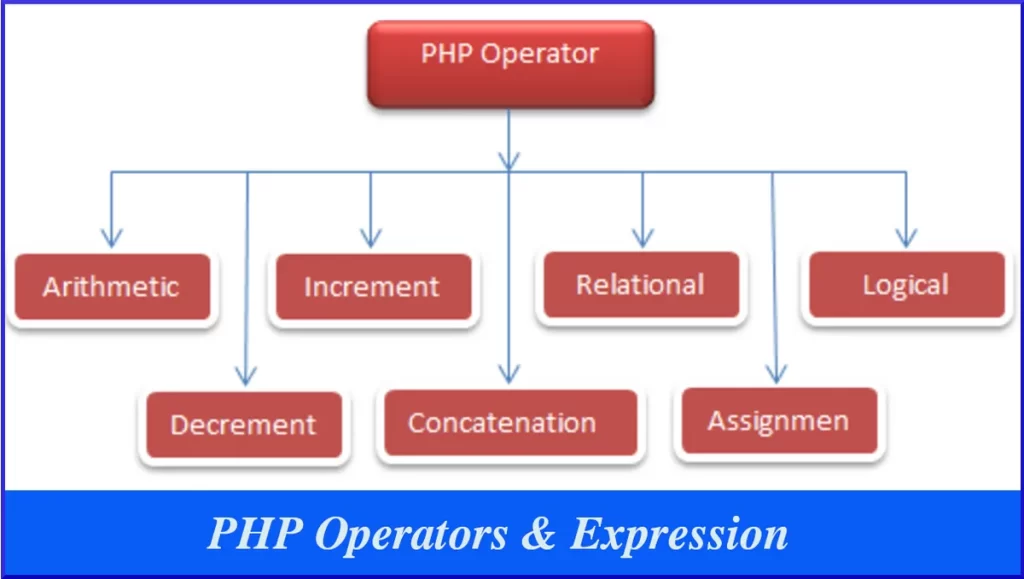
Arithmetic operator in PHP:
Arithmetic operators Example like: +, -, *, /, % (modulus), ** (exponentiation)
Operator | Name | Example | Explanation |
---|---|---|---|
+ | Addition | $a + $b | Sum of operands |
– | Subtraction | $a – $b | Difference of operands |
* | Multiplication | $a * $b | Product of operands |
/ | Division | $a / $b | Quotient of operands |
% | Modulus | $a % $b | Remainder of operands |
** | Exponentiation | $a ** $b | $a raised to the power $b |
Assignment operator in PHP:
Assignment operators: =, +=, -=, *=, /=, %=, .= (concatenation assignment)
Operator | Name | Example | Explanation |
---|---|---|---|
= | Assign | $a = $b | The value of right operand is assigned to the left operand. |
+= | Add then Assign | $a += $b | Addition same as $a = $a + $b |
-= | Subtract then Assign | $a -= $b | Subtraction same as $a = $a – $b |
*= | Multiply then Assign | $a *= $b | Multiplication same as $a = $a * $b |
/= | Divide then Assign (quotient) | $a /= $b | Find quotient same as $a = $a / $b |
%= | Divide then Assign (remainder) | $a %= $b | Find remainder same as $a = $a % $b |
Comparison operators:
Comparison operators: ==, === (identical), !=, !== (not identical), <, >, <=, >=
Operator | Name | Example | Explanation |
---|---|---|---|
== | Equal | $a == $b | Return TRUE if $a is equal to $b |
=== | Identical | $a === $b | Return TRUE if $a is equal to $b, and they are of same data type |
!== | Not identical | $a !== $b | Return TRUE if $a is not equal to $b, and they are not of same data type |
!= | Not equal | $a != $b | Return TRUE if $a is not equal to $b |
<> | Not equal | $a <> $b | Return TRUE if $a is not equal to $b |
< | Less than | $a < $b | Return TRUE if $a is less than $b |
> | Greater than | $a > $b | Return TRUE if $a is greater than $b |
<= | Less than or equal to | $a <= $b | Return TRUE if $a is less than or equal $b |
>= | Greater than or equal to | $a >= $b | Return TRUE if $a is greater than or equal $b |
<=> | Spaceship | $a <=>$b | Return -1 if $a is less than $b Return 0 if $a is equal $b Return 1 if $a is greater than $b |
Logical operator:
Logical operators: && (and), || (or), ! (not)
Operator | Name | Example | Explanation |
---|---|---|---|
and | And | $a and $b | Return TRUE if both $a and $b are true |
Or | Or | $a or $b | Return TRUE if either $a or $b is true |
xor | Xor | $a xor $b | Return TRUE if either $ or $b is true but not both |
! | Not | ! $a | Return TRUE if $a is not true |
&& | And | $a && $b | Return TRUE if either $a and $b are true |
|| | Or | $a || $b | Return TRUE if either $a or $b is true |
Increment and Decrement operator:
Increment/Decrement operators: ++ (increment), — (decrement)
Name | Operator |
Pre-increment | ++$x |
Post-Increment | $x- – |
Pre-decrement | –$x |
Post-decrement | $x- – |
Bitwise operator:
Bitwise operators: & (and), | (or), ^ (xor), ~ (not), << (left shift), >> (right shift)
Operator | Name | Example | Explanation |
---|---|---|---|
& | And | $a & $b | Bits that are 1 in both $a and $b are set to 1, otherwise 0. |
| | Or (Inclusive or) | $a | $b | Bits that are 1 in either $a or $b are set to 1 |
^ | Xor (Exclusive or) | $a ^ $b | Bits that are 1 in either $a or $b are set to 0. |
~ | Not | ~$a | Bits that are 1 set to 0 and bits that are 0 are set to 1 |
<< | Shift left | $a << $b | Left shift the bits of operand $a $b steps |
>> | Shift right | $a >> $b | Right shift the bits of $a operand by $b number of places |