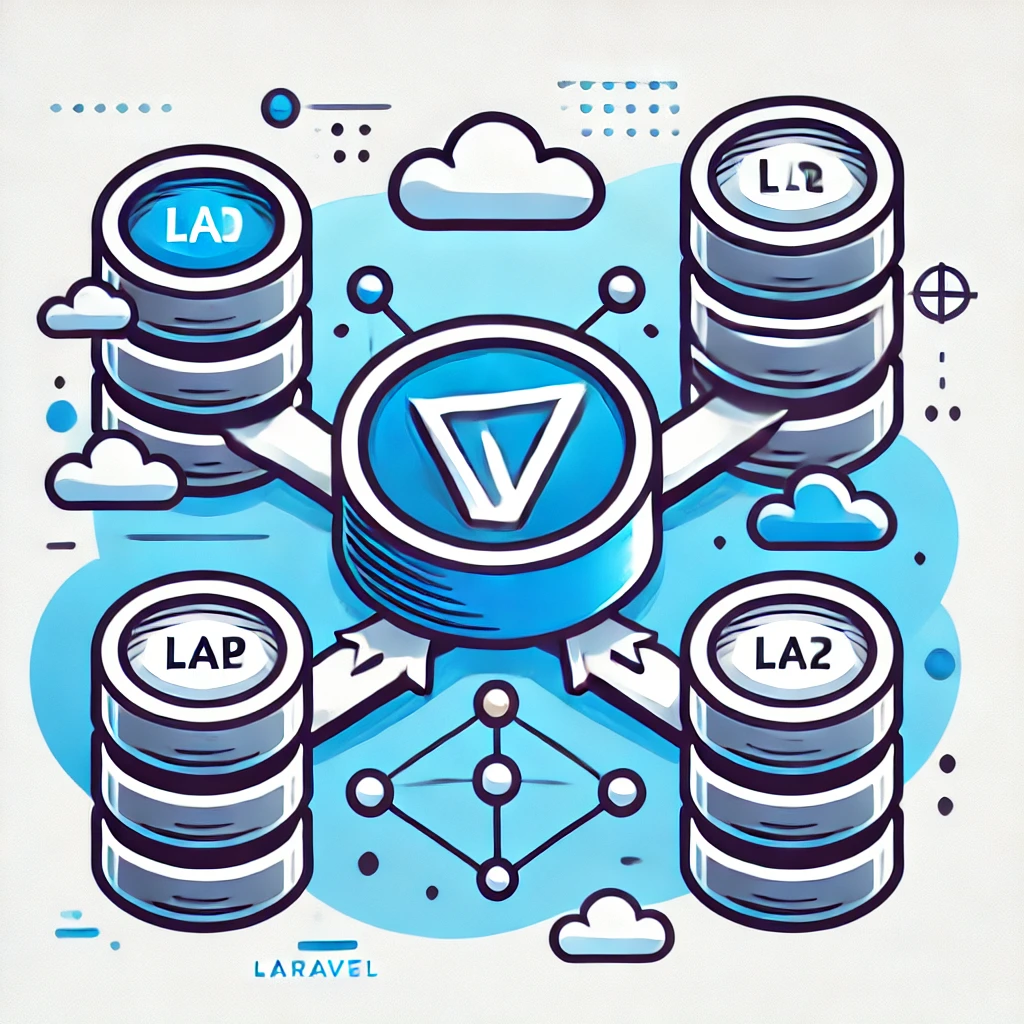
To configure multiple databases in Laravel, you’ll need to modify your .env
file as well as the config/database.php
file to manage the connections.
Step 1: Update the .env
file
In your .env
file, define the settings for both databases. You can have a default connection as well as a second connection with a new prefix.
Example .env
file:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=default_database
DB_USERNAME=root
DB_PASSWORD=password
DB_SECOND_CONNECTION=mysql
DB_SECOND_HOST=127.0.0.1
DB_SECOND_PORT=3306
DB_SECOND_DATABASE=second_database
DB_SECOND_USERNAME=root
DB_SECOND_PASSWORD=password
Step 2: Configure config/database.php
Now, go to the config/database.php
file and configure the connections. Laravel uses the default connection for the database interactions unless specified otherwise. To add multiple database connections, follow these steps:
In the connections
array inside config/database.php
, add a new connection for the second database like this:
'connections' => [
'mysql' => [
'driver' => 'mysql',
'host' => env('DB_HOST', '127.0.0.1'),
'port' => env('DB_PORT', '3306'),
'database' => env('DB_DATABASE', 'forge'),
'username' => env('DB_USERNAME', 'forge'),
'password' => env('DB_PASSWORD', ''),
'unix_socket' => env('DB_SOCKET', ''),
'charset' => 'utf8mb4',
'collation' => 'utf8mb4_unicode_ci',
'prefix' => '',
'strict' => true,
'engine' => null,
],
'mysql2' => [
'driver' => 'mysql',
'host' => env('DB_SECOND_HOST', '127.0.0.1'),
'port' => env('DB_SECOND_PORT', '3306'),
'database' => env('DB_SECOND_DATABASE', 'forge'),
'username' => env('DB_SECOND_USERNAME', 'forge'),
'password' => env('DB_SECOND_PASSWORD', ''),
'unix_socket' => env('DB_SECOND_SOCKET', ''),
'charset' => 'utf8mb4',
'collation' => 'utf8mb4_unicode_ci',
'prefix' => '',
'strict' => true,
'engine' => null,
],
],
Step 3: Using Multiple Databases in Your Code
Now that you have multiple connections, you can specify which connection to use in your models, queries, or migrations.
For example, if you want to use the second database connection in a query:
$users = DB::connection('mysql2')->select('SELECT * FROM users');
Or if you want to specify a connection for a particular model:
class User extends Model
{
protected $connection = 'mysql2'; // Use the second database connection
}
Step 4: Running Migrations
To run migrations for different connections, you can specify the connection like this:
php artisan migrate --database=mysql2
This will run the migrations on the second database connection.
By following these steps, you can easily configure and use multiple databases in Laravel.