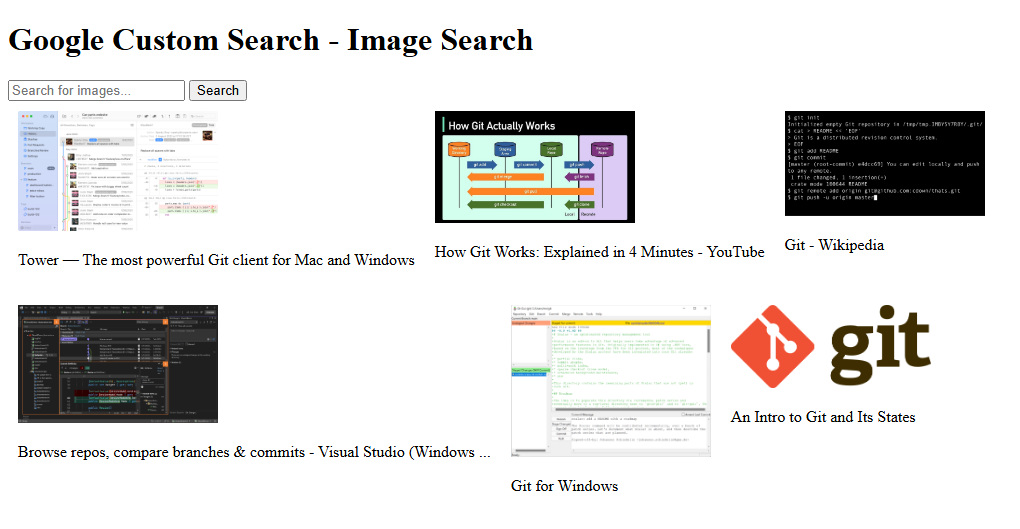
Creating a Google Search API involves several steps, mostly centered around accessing Google’s Custom Search JSON API, which lets you develop websites and applications to retrieve and display search results from Google Custom Search programmatically. Here’s how you can set it up step-by-step:
Step 1: Set Up a Google Cloud Project
- Create a Google Cloud account: Go to the Google Cloud Console (https://console.cloud.google.com/) and sign up or log in.
- Create a new project: Click on the project drop-down menu at the top of the dashboard, then click on “New Project”. Name your project and set up billing (required for using the Custom Search JSON API).
Step 2: Enable Custom Search API
- Navigate to the API Library: In the Google Cloud Console, go to the “APIs & Services > Library”.
- Search for “Custom Search API” and select it.
- Enable the API for your project.
Step 3: Set Up a Custom Search Engine
- Go to Google Custom Search Engine page (https://cse.google.com/cse/).
- Create a new search engine:
- Click on “Add”.
- In the “Sites to search” field, enter the websites you want the search engine to search across.
- Specify further settings such as language and give your search engine a name.
- Click “Create”.
3. Modify and configure your search engine:
- Once created, you can adjust the search engine to search the entire web or only the sites you have specified.
- Customize the search features as needed.
Step 4: Get Your API Key and Search Engine ID
- API Key:
- Go back to the Google Cloud Console.
- Navigate to “Credentials” on the sidebar.
- Click on “Create Credentials” and select “API key”. This key will be used in your API requests.
2. Search Engine ID:
- Go to your Custom Search Engine’s control panel.
- Find the “Search engine ID” under the “Basics” tab.
Step 5: Implement the API
- Use the API in your application:
- You can start making HTTP requests to the API using the following endpoint:
https://www.googleapis.com/customsearch/v1?q={QUERY}&cx={SEARCH_ENGINE_ID}&key={API_KEY}
- Replace
{QUERY}
with your search query,{SEARCH_ENGINE_ID}
with your Search Engine ID, and{API_KEY}
with your API key.
Step 6: Handle the JSON Response
- The API will return a JSON response containing the search results. Parse this JSON to display the results in your application as needed.
Step 7: Optimize and Secure
- Limit API key usage: Restrict the API key in Google Cloud Console by setting application restrictions and API restrictions to prevent unauthorized use.
- Monitor usage: Regularly check the usage reports in Google Cloud Console to monitor the API usage and adjust as necessary.
Example API Call Using Python
Here’s a simple Python example to use the Google Custom Search JSON API:
import requests
# Replace 'your_api_key' and 'your_cse_id' with your API Key and Custom Search Engine ID
API_KEY = 'your_api_key'
SEARCH_ENGINE_ID = 'your_cse_id'
QUERY = 'example search'
url = f"https://www.googleapis.com/customsearch/v1?q={QUERY}&cx={SEARCH_ENGINE_ID}&key={API_KEY}"
response = requests.get(url)
search_results = response.json()
# Print titles and URLs of results
for result in search_results['items']:
print(result['title'], result['link'])
This setup allows you to integrate Google Search capabilities into your applications and handle search queries customized to your requirements.
Example PHP Code to Call Google Custom Search API
Here’s how you can make an API call to the Google Custom Search JSON API using PHP. This example will use curl
, a common way to make HTTP requests in PHP.
In another Example, PHP Code to Call Google Custom Search API
<?php
// Your Google API key and Custom Search Engine ID
$apiKey = 'your_api_key';
$searchEngineId = 'your_search_engine_id';
$query = 'example search';
// Create the URL for the request
$url = "https://www.googleapis.com/customsearch/v1?q=" . urlencode($query) . "&cx=$searchEngineId&key=$apiKey";
// Initialize cURL session
$curl = curl_init();
// Set cURL options
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
curl_setopt($curl, CURLOPT_HEADER, false);
// Execute cURL session and get the response
$response = curl_exec($curl);
// Close cURL session
curl_close($curl);
// Decode the JSON response
$results = json_decode($response, true);
// Check if the items key exists to handle errors or empty results
if (isset($results['items'])) {
foreach ($results['items'] as $item) {
echo "Title: " . $item['title'] . "\n";
echo "URL: " . $item['link'] . "\n\n";
}
} else {
echo "No results found or error occurred.";
}
?>
Key Points:
- cURL Initialization: Start a new cURL session and set the URL of the Google API.
- cURL Options: Configure options for the cURL session:
CURLOPT_URL
sets the URL to fetch.CURLOPT_RETURNTRANSFER
tells cURL to return the response as a string instead of outputting it directly.CURLOPT_HEADER
set to false to exclude headers in the output (only the body is returned).
- Execute and Close cURL: The API response is fetched and the cURL session is closed.
- Decode JSON: Convert the JSON response into a PHP array.
- Handling Results: Iterate through the results if available and print titles and URLs.
Usage Tips:
- Replace
'your_api_key'
and'your_search_engine_id'
with your actual API key and search engine ID. - Error handling and security enhancements, such as checking for cURL errors (
curl_error()
) and restricting API key usage via the Google Cloud Console, are recommended for production environments.