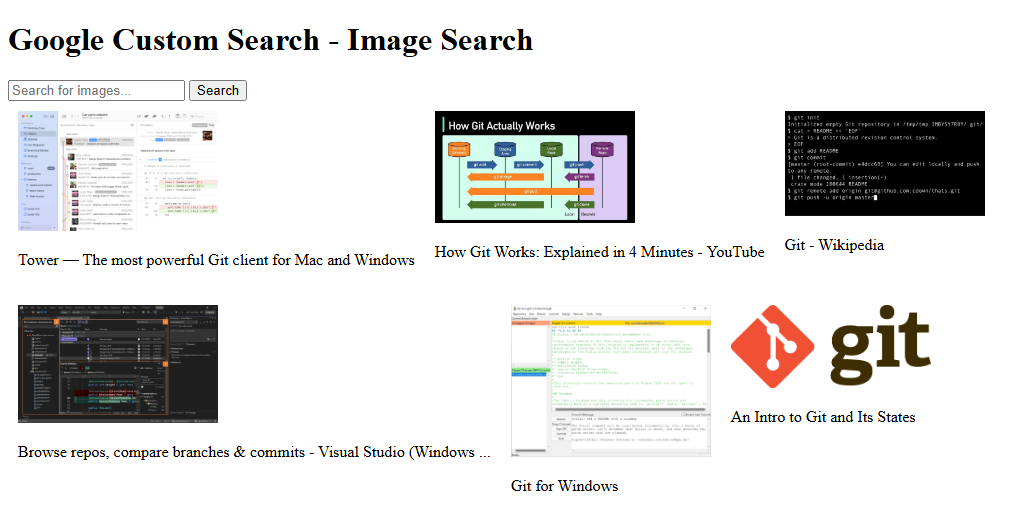
Building an image search engine using the Google Custom Search API and PHP involves several steps. Here is a detailed guide:
Prerequisites
- Google Cloud Account: Make sure you have a Google account. Sign up for a Google Cloud Platform account if you don’t already have one.
- Custom Search Engine (CSE): Create a Custom Search Engine on Google.
- Google API Key: Get an API key to access the Google Custom Search API.
- PHP Environment: Ensure you have a working PHP environment (local server, or use a hosting service).
Step 1: Create a Custom Search Engine
- Go to the Google Custom Search Engine.
- Click “Add” to create a new search engine.
- Define the sites to search (you can leave this blank to search the whole web).
- Click on “Create”. You’ll get a Search Engine ID. Make note of it.
Step 2: Get Google API Key
- Go to the Google Developers Console.
- Create a new project (or use an existing one).
- Enable the Custom Search JSON API in your project.
- Go to Credentials and create a new API key. Make note of this key.
Step 3: PHP Code for Image Search
Now, we will write the PHP code to perform the image search.
Sample PHP Code:
<?php
// Check if the form has been submitted
if (isset($_GET['search'])) {
// Your Google API key and Custom Search Engine ID
$apiKey = 'your_api_key';
$searchEngineId = 'your_search_engine_id';
$query = $_GET['search'];
// Create the URL for the request
$url = "https://www.googleapis.com/customsearch/v1?q=" . urlencode($query) . "&cx=$searchEngineId&key=$apiKey&searchType=image";
// Initialize cURL session
$curl = curl_init();
// Set cURL options
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
curl_setopt($curl, CURLOPT_HEADER, false);
// Execute cURL session and get the response
$response = curl_exec($curl);
// Close cURL session
curl_close($curl);
// Decode the JSON response
$results = json_decode($response, true);
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Image Search</title>
</head>
<body>
<h1>Google Custom Search - Image Search</h1>
<form action="" method="get">
<input type="text" name="search" placeholder="Search for images...">
<button type="submit">Search</button>
</form>
<?php
if (!empty($results['items'])) {
echo "<div style='display: flex; flex-wrap: wrap;'>";
foreach ($results['items'] as $item) {
echo "<div style='margin: 10px;'>";
echo "<img src='" . $item['link'] . "' alt='" . htmlspecialchars($item['title']) . "' style='width: 200px; height: auto;'><br>";
echo "<p>" . htmlspecialchars($item['title']) . "</p>";
echo "</div>";
}
echo "</div>";
} elseif (isset($_GET['search'])) {
echo "<p>No results found or error occurred.</p>";
}
?>
</body>
</html>
Step 4: Replace API Key and Search Engine ID
- Replace
YOUR_GOOGLE_API_KEY
with your actual Google API Key. - Replace
YOUR_SEARCH_ENGINE_ID
with your Custom Search Engine ID.
Step 5: Run the PHP Script
- Save the PHP code in a file (e.g.,
image-search.php
). - Place the file on your server or local environment.
- Access the file in your browser (e.g.,
http://localhost/image-search.php
).
How It Works:
- HTML Form: A simple HTML form is provided for users to enter their search queries.
- Image Search Handling in PHP:
- The script checks if there is a
GET
request with the search parameter. - It appends
&searchType=image
to the query string in the API URL to specify that only images should be returned.
3. Displaying Results:
- The results are looped through and each image is displayed with its title. Images are displayed in a flexible layout.
- HTML
img
tags are used to display images, and PHPhtmlspecialchars()
is used to escape titles for safe HTML output.