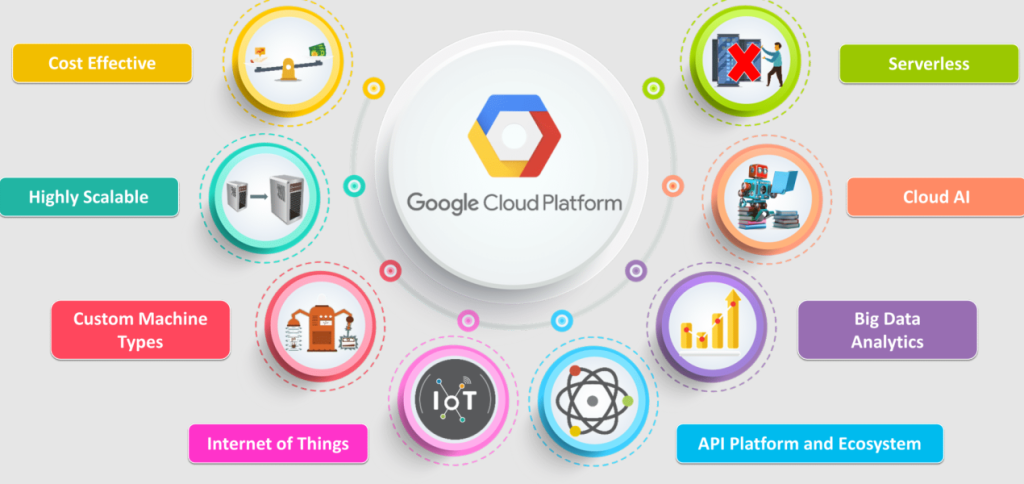
Google Cloud AI Platform is a suite of machine learning and artificial intelligence services offered by Google Cloud to help developers, data scientists, and businesses build, train, and deploy machine learning models at scale. It provides tools for automating the entire machine-learning lifecycle, including data preparation, model development, training, deployment, and monitoring. The platform integrates seamlessly with other Google Cloud services like BigQuery, Google Kubernetes Engine (GKE), and TensorFlow, enabling users to leverage powerful infrastructure and scalable resources. Google Cloud AI Platform supports a wide range of use cases, including natural language processing (NLP) for chatbots and sentiment analysis, image and video analysis for computer vision tasks, predictive analytics for business forecasting, and recommendation systems for personalized content. It is used across industries like healthcare, retail, finance, and manufacturing to derive insights from large datasets, optimize processes, and improve decision-making with AI-powered solutions.
What is Google Cloud AI Platform?
Google Cloud AI Platform provides a unified platform for building and operationalizing machine learning models. It offers services for data preparation, model training, hyperparameter tuning, model deployment, and monitoring. Designed to handle machine learning workflows of any complexity, it supports popular frameworks like TensorFlow, PyTorch, and Scikit-learn.
Key Characteristics:
- Scalable Infrastructure: Leverages Google Cloud’s powerful computing and storage resources.
- End-to-End AI Lifecycle Management: Covers every aspect of AI and ML workflows, from data preprocessing to production deployment.
- Framework Compatibility: Supports multiple ML frameworks and APIs for seamless integration.
Top 10 Use Cases of Google Cloud AI Platform
- Image Recognition: Train and deploy deep learning models for image classification and object detection in various domains, such as healthcare and retail.
- Natural Language Processing (NLP): Build models for sentiment analysis, language translation, text summarization, and chatbot development.
- Recommendation Systems: Design personalized recommendation engines for e-commerce platforms, streaming services, and content delivery networks.
- Predictive Analytics: Leverage historical data to predict trends, customer behavior, or market outcomes in sectors like finance and marketing.
- Fraud Detection: Develop machine learning models to detect fraudulent activities in real-time, particularly in banking and insurance.
- Customer Segmentation: Use clustering and classification techniques to segment customers for targeted marketing strategies.
- Speech Recognition: Create speech-to-text models for applications like virtual assistants, transcription services, and call center analytics.
- Healthcare Diagnostics: Train AI models to analyze medical images, predict disease outcomes, or optimize treatment plans.
- Time Series Forecasting: Build predictive models for energy consumption, stock prices, or weather patterns.
- Supply Chain Optimization: Use AI models to optimize logistics, inventory management, and demand forecasting.
Features of Google Cloud AI Platform
- AI Platform Notebooks: Integrated Jupyter notebooks for developing and testing machine learning workflows with minimal setup.
- Scalable Training: Support for distributed training across multiple GPUs and TPUs, reducing training time for large models.
- Hyperparameter Tuning: Automated tuning of model parameters to achieve optimal performance.
- Model Serving: Scalable and secure model deployment with AI Platform Prediction, providing REST API endpoints.
- AutoML: Automated model building for image classification, text analysis, and structured data without extensive programming knowledge.
- BigQuery Integration: Seamlessly integrates with BigQuery for large-scale data analysis and training.
- End-to-End Security: Provides security features like data encryption, IAM policies, and compliance with industry standards.
- Multi-Framework Support: Works with popular ML frameworks like TensorFlow, PyTorch, and XGBoost.
- Explainable AI: Tools for interpreting and understanding machine learning model predictions.
- MLOps Integration: Comprehensive support for CI/CD pipelines, monitoring, and logging for production-ready machine learning.
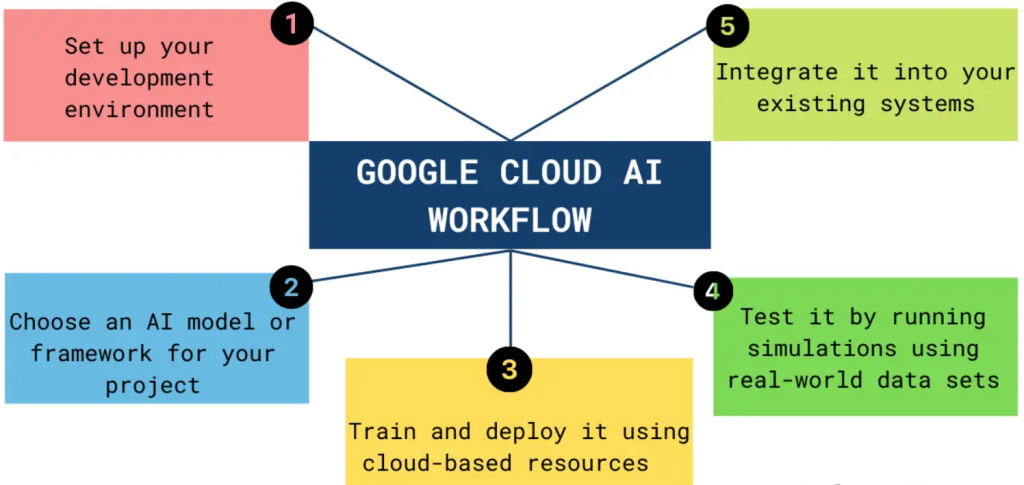
How Google Cloud AI Platform Works and Architecture
- Data Ingestion and Preparation: Use tools like Google Cloud Storage and BigQuery to ingest and preprocess large datasets.
- Model Development: Develop machine learning models using AI Platform Notebooks or your preferred framework and programming language.
- Model Training: Train models on Google Cloud’s distributed infrastructure using CPUs, GPUs, or TPUs for faster results.
- Hyperparameter Optimization: Optimize model performance with built-in hyperparameter tuning features.
- Model Deployment: Deploy models as REST APIs via AI Platform Prediction or use containerized deployment on Kubernetes Engine.
- Monitoring and Maintenance: Track model performance in production with monitoring and logging tools, ensuring consistency and reliability.
How to Install Google Cloud AI Platform
To use Google Cloud AI Platform programmatically, you need to set up the Google Cloud SDK and install the necessary Python packages for interacting with Google Cloud services. The following steps will guide you through the installation process to access and use AI Platform for training and deploying machine learning models:
1. Set Up a Google Cloud Account
First, make sure you have a Google Cloud account. If you don’t have one, you can sign up at Google Cloud.
- After creating your account, set up a Google Cloud project and enable billing.
2. Install Google Cloud SDK
Google Cloud AI Platform requires the Google Cloud SDK to interact with your Google Cloud resources. Install the SDK by following the instructions for your operating system.
- For macOS/Linux:
curl https://sdk.cloud.google.com | bash
- For Windows: Download the installer from the Google Cloud SDK website and follow the instructions.
3. Authenticate Your Account
Once the SDK is installed, authenticate your Google Cloud account:
gcloud auth login
This will open a browser window where you can log in with your Google account.
4. Set Your Google Cloud Project
Set the active Google Cloud project that you will use for AI Platform:
gcloud config set project YOUR_PROJECT_ID
Replace YOUR_PROJECT_ID
with the actual ID of your Google Cloud project.
5. Install Python Client Libraries
To interact with Google Cloud AI Platform programmatically using Python, you need to install the relevant libraries. Use pip
to install the Google Cloud AI Platform Python SDK:
pip install google-cloud-ai-platform
6. Install Additional Dependencies (Optional)
If you are using other Google Cloud services (e.g., storage for datasets), you might also need the google-cloud-storage
library:
pip install google-cloud-storage
You may also need other libraries depending on the specific services you intend to use (e.g., for TensorFlow, scikit-learn, etc.).
7. Create and Train a Model on the Google Cloud AI Platform
Once the setup is complete, you can start using AI Platform to train and deploy machine learning models. Here’s an example of training a model on the Google Cloud AI Platform using Python.
from google.cloud import aiplatform
# Set the project ID and region
project_id = "YOUR_PROJECT_ID"
region = "us-central1" # or the region you are using
# Initialize the AI Platform client
aiplatform.init(project=project_id, location=region)
# Define the model training job
training_job = aiplatform.CustomJob(
display_name="my_model_training",
worker_pool_specs=[{
"machine_spec": {"machine_type": "n1-standard-4"},
"replica_count": 1,
"python_package_spec": {
"executor_image_uri": "gcr.io/cloud-aiplatform/training/tf2-cpu.2-3:latest",
"package_uris": ["gs://your_bucket/your_package.tar.gz"],
"python_module": "your_training_module"
}
}]
)
# Run the training job
training_job.run(sync=True)
Replace YOUR_PROJECT_ID
with your Google Cloud project ID and modify the job specifications accordingly. This example demonstrates training a TensorFlow model, but you can adapt it for other machine learning frameworks like scikit-learn, PyTorch, or XGBoost.
8. Deploying the Model
After training the model, you can deploy it to AI Platform for predictions. Here’s how to deploy the trained model:
# Deploy the trained model
endpoint = training_job.run(sync=True).endpoint
# Use the endpoint to make predictions
prediction = endpoint.predict(instances=[...]) # Provide your input data
print(prediction)
9. Monitor and Manage Models
Once deployed, you can monitor and manage your models using Google Cloud Console or by interacting programmatically with the AI Platform API.
Basic Tutorials of Google Cloud AI Platform: Getting Started
Step 1: Create a Notebook Instance
Use AI Platform Notebooks to create a Jupyter Notebook for development:
- Navigate to AI Platform > Notebooks in the Google Cloud Console.
- Click Create Instance and select a framework (e.g., TensorFlow, PyTorch).
Step 2: Load Data
Import data from Google Cloud Storage or BigQuery into the notebook.
from google.cloud import storage
client = storage.Client()
bucket = client.get_bucket('your-bucket-name')
blob = bucket.blob('your-data-file.csv')
blob.download_to_filename('local-file.csv')
Step 3: Train a Model
Train a machine learning model using your preferred framework.
from sklearn.ensemble import RandomForestClassifier
model = RandomForestClassifier()
model.fit(X_train, y_train)
Step 4: Deploy the Model
Deploy the trained model as a REST API:
gcloud ai-platform models create my_model
gcloud ai-platform versions create v1 --model my_model --origin gs://my-bucket/model-dir --runtime-version 2.1
Step 5: Test and Monitor
Test the deployed model using the provided REST endpoint and monitor performance using Google Cloud tools.