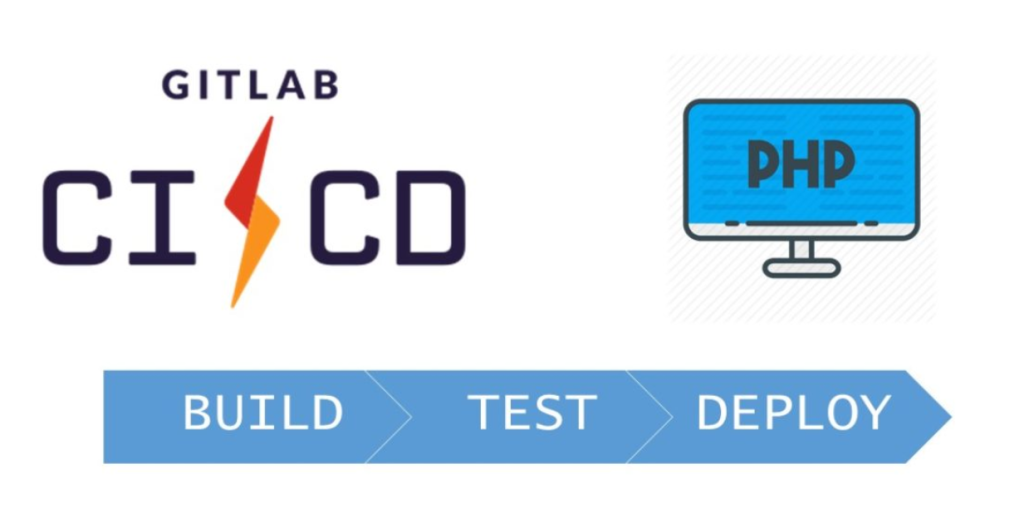
GitLab CI/CD is a built-in feature of GitLab, a popular web-based Git repository manager, that enables continuous integration and continuous delivery (CI/CD) automation for software development projects. GitLab CI/CD allows developers to automatically build, test, and deploy applications directly from their GitLab repositories. By defining pipeline configurations in a .gitlab-ci.yml
file, users can set up workflows for automating various tasks such as code compilation, running unit tests, security checks, deployment, and monitoring. GitLab CI/CD integrates with GitLab’s version control, issue tracking, and code review features, making it an all-in-one solution for modern DevOps practices.
GitLab CI/CD is used across various stages of software development. In continuous integration, it automates the process of running tests and building applications whenever new code is committed, ensuring that bugs and errors are detected early. In continuous delivery, GitLab automates the deployment of applications to different environments, ensuring rapid and reliable delivery of new features or bug fixes. It is also used for automating testing, including unit tests, integration tests, and security scans, which helps ensure that code meets quality and security standards before it is merged or deployed. Additionally, GitLab CI/CD is valuable in DevOps environments, enabling collaboration between development and operations teams, streamlining the release cycle, and reducing manual intervention in the deployment process.
What is GitLab CI/CD?
GitLab CI/CD is a built-in feature of GitLab that allows developers to define, manage, and execute CI/CD pipelines directly within their Git repositories. Using the .gitlab-ci.yml
file, developers can create pipelines to automate code testing, building, and deployment across multiple environments. It supports various programming languages, frameworks, and cloud providers, making it a versatile tool for DevOps practices.
Key Characteristics of GitLab CI/CD:
- End-to-End Integration: Works natively with GitLab, eliminating the need for external CI/CD tools.
- Scalability: Handles workloads for small teams and large enterprises.
- Ease of Use: Configured through a YAML file in the project repository.
- Flexibility: Supports various CI/CD workflows, from simple builds to complex multi-environment deployments.
Top 10 Use Cases of GitLab CI/CD
- Automated Code Testing
- Runs unit, integration, and regression tests to ensure the quality and functionality of the code.
- Continuous Integration
- Automates the process of integrating code changes into the main branch and ensures compatibility through build verification.
- Continuous Delivery
- Automates deployment to staging environments, allowing teams to release software quickly and reliably.
- Continuous Deployment
- Automatically deploys code to production environments after passing predefined quality checks.
- Microservices Deployment
- Manages independent pipelines for multiple microservices in a single project.
- Infrastructure as Code (IaC)
- Automates the provisioning and management of infrastructure using tools like Terraform and Ansible.
- Docker Image Builds
- Builds and pushes Docker images to container registries for containerized applications.
- Mobile Application CI/CD
- Automates the building, testing, and deployment of iOS and Android apps, including code signing.
- Multi-Cloud Deployments
- Deploys applications to multiple cloud providers, including AWS, Google Cloud, and Azure.
- DevSecOps Pipelines
- Integrates security scans, such as SAST, DAST, and dependency scanning, into the CI/CD pipeline to ensure compliance and prevent vulnerabilities.
Features of GitLab CI/CD
- Integrated CI/CD – Built directly into GitLab, providing a seamless experience for code hosting and automation.
- Pipeline as Code – Uses a
.gitlab-ci.yml
file to define and manage pipelines. - Multi-Stage Pipelines – Allows complex workflows with stages such as build, test, and deploy.
- Parallel Builds – Executes multiple jobs simultaneously to reduce pipeline execution times.
- Environment Management – Supports managing different environments like staging, production, and testing.
- Docker Integration – Natively integrates with Docker for containerized application builds and deployments.
- Advanced Security – Includes features like SAST, DAST, and secret detection to secure applications.
- Auto DevOps – Automates pipeline creation and deployment for applications following GitLab’s best practices.
- Monitoring and Feedback – Tracks pipeline performance and logs for better visibility.
- Role-Based Access Control (RBAC) – Provides granular access control to secure projects and pipelines.
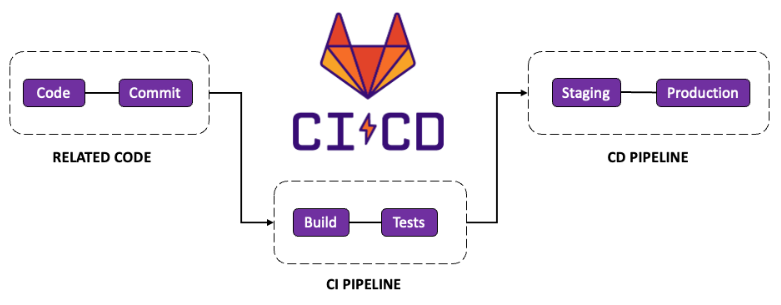
How GitLab CI/CD Works and Architecture
1. Configuration with .gitlab-ci.yml
The pipeline configuration is defined in a YAML file stored in the root directory of the repository. This file specifies:
- Jobs: Tasks to be executed (e.g., building, testing, deploying).
- Stages: Groups of jobs (e.g., build, test, deploy).
- Scripts: Commands to be run for each job.
2. Pipelines
A pipeline is triggered whenever code is pushed to the repository. Pipelines consist of multiple stages executed sequentially or in parallel.
3. Runners
GitLab CI/CD uses runners to execute pipeline jobs. Runners can be:
- Shared Runners: Managed by GitLab for shared use across projects.
- Specific Runners: Dedicated runners for specific projects or teams.
4. Artifacts and Caching
- Artifacts: Files generated during a pipeline, such as build outputs or test reports.
- Caching: Speeds up pipelines by caching dependencies and build artifacts.
5. Deployment and Monitoring
Deployed applications can be monitored using GitLab’s built-in metrics and logging integrations.
How to Install GitLab CI/CD
GitLab CI/CD is built into GitLab, meaning you don’t need to install a separate tool for it. To use GitLab CI/CD, you simply need a GitLab repository and a .gitlab-ci.yml
file to define the pipeline configuration. However, setting up a GitLab CI/CD pipeline requires some steps to configure your repository and define the automation workflows. Below is a step-by-step guide for integrating GitLab CI/CD into your project:
1. Create a GitLab Account
- First, if you don’t already have one, sign up for a GitLab account at GitLab.com.
- You can also use GitLab Self-Managed if you are hosting GitLab on your own server.
2. Create a GitLab Repository
- After signing up, create a new project (repository) in GitLab by clicking on “New Project.”
- Push your code to GitLab if it’s not already hosted there.
3. Create .gitlab-ci.yml
File
- In the root directory of your project, create a
.gitlab-ci.yml
file. This file defines your CI/CD pipeline configuration, such as build, test, and deploy stages.
Example of a simple .gitlab-ci.yml
file:
stages:
- build
- test
- deploy
build:
stage: build
script:
- echo "Building the application"
- make build # Replace with actual build command for your project
test:
stage: test
script:
- echo "Running tests"
- make test # Replace with actual test command for your project
deploy:
stage: deploy
script:
- echo "Deploying the application"
- make deploy # Replace with actual deploy command for your project
only:
- master # Only deploy when changes are pushed to the master branch
In this configuration:
- Stages: Defines the stages of your pipeline (build, test, deploy).
- Jobs: Each job corresponds to a stage and contains the scripts to run during that stage.
- Script: Commands to run for each job.
- Only: Ensures the deploy job only runs for the
master
branch.
4. Push .gitlab-ci.yml
to GitLab
After creating your .gitlab-ci.yml
file, push it to your GitLab repository:
git add .gitlab-ci.yml
git commit -m "Add GitLab CI/CD configuration"
git push origin main
5. GitLab Detects the Pipeline
Once you push the .gitlab-ci.yml
file, GitLab automatically detects the file and starts the pipeline. You can monitor the status of the pipeline directly in the CI/CD > Pipelines section of your GitLab project.
- GitLab will trigger the pipeline automatically based on your commit.
- The pipeline will run through the stages you defined (build, test, deploy).
6. Monitoring the Pipeline
- In your GitLab repository, go to CI/CD > Pipelines to monitor the progress of your pipeline.
- You can see logs for each job (build, test, deploy), check for errors, and see if all jobs succeed or fail.
7. Advanced Configuration (Optional)
You can extend your .gitlab-ci.yml
file with more advanced features:
- Caching dependencies: To speed up your builds by caching dependencies.
- Variables: To define environment variables like API keys or credentials.
- Docker Integration: To build and test applications inside Docker containers.
- Triggers: To trigger pipelines based on other conditions like tags, or manual approval.
Example of caching dependencies:
cache:
paths:
- node_modules/
8. Integrate with Deployment Tools (Optional)
If you want to deploy your app automatically to a cloud provider (like AWS, Azure, Heroku), you can add deployment steps to your .gitlab-ci.yml
file.
Example of deployment to Heroku:
deploy:
stage: deploy
script:
- echo "Deploying to Heroku"
- git remote add heroku https://git.heroku.com/your-app.git
- git push heroku master
only:
- master
Basic Tutorials of GitLab CI/CD: Getting Started
Step 1: Create a Repository
- Create a new project in GitLab.
- Clone the repository to your local machine:
git clone https://gitlab.com/username/project.git
Step 2: Add a .gitlab-ci.yml
File
- Add the following example
.gitlab-ci.yml
file to your repository:
stages:
- build
- test
- deploy
build-job:
stage: build
script:
- echo "Building the application..."
test-job:
stage: test
script:
- echo "Running tests..."
deploy-job:
stage: deploy
script:
- echo "Deploying the application..."
- Commit and push the file:
git add .gitlab-ci.yml
git commit -m "Add CI/CD pipeline"
git push origin main
Step 3: Monitor the Pipeline
- Go to the CI/CD > Pipelines section in your GitLab project.
- Monitor the pipeline’s progress and view logs for each job.
Step 4: Enhance the Pipeline
- Add more stages and jobs as needed.
- Integrate testing tools, security scans, or deployment scripts.