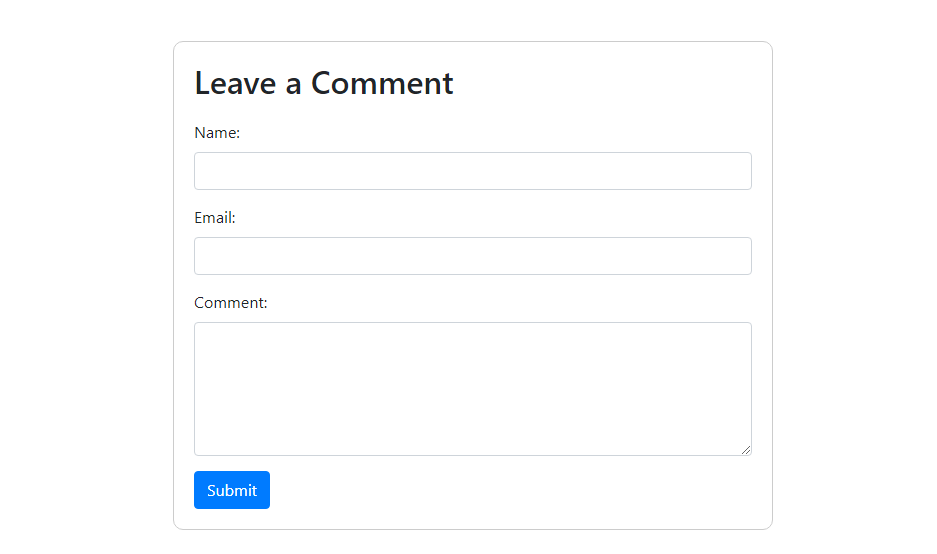
Creating a Laravel comment form with an attractive style involves a few steps: setting up the form in a Blade template, creating a controller to handle the form submission, and styling the form using CSS. Here’s a step-by-step guide to achieve this.
Step 1: Setting Up Laravel Project
First, ensure you have a Laravel project set up. If not, you can create a new one using the following command:
composer create-project --prefer-dist laravel/laravel comment_form
Step 2: Creating a Comment Model and Migration
Create a Comment
model and a migration file:
php artisan make:model Comment -m
In the migration file (database/migrations/xxxx_xx_xx_create_comments_table.php
), define the necessary fields:
public function up()
{
Schema::create('comments', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->string('email');
$table->text('comment');
$table->timestamps();
});
}
Run the migration to create the table:
php artisan migrate
Step 3: Creating a CommentController
Create a controller to handle form submission:
php artisan make:controller CommentController
In the CommentController.php
file, add the following methods:
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Comment;
class CommentController extends Controller
{
public function store(Request $request)
{
$request->validate([
'name' => 'required',
'email' => 'required|email',
'comment' => 'required',
]);
Comment::create($request->all());
return back()->with('success', 'Comment submitted successfully!');
}
}
Step 4: Creating the Comment Form Blade Template
Create a Blade template for the comment form, e.g., resources/views/comment_form.blade.php
:
<!DOCTYPE html>
<html>
<head>
<title>Laravel Comment Form</title>
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
<style>
.comment-form {
max-width: 600px;
margin: 50px auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 10px;
}
.comment-form h2 {
margin-bottom: 20px;
}
.comment-form .form-group {
margin-bottom: 15px;
}
.comment-form button {
background-color: #007bff;
color: #fff;
}
</style>
</head>
<body>
<div class="container">
<div class="comment-form">
<h2>Leave a Comment</h2>
@if(session('success'))
<div class="alert alert-success">
{{ session('success') }}
</div>
@endif
<form action="{{ route('comments.store') }}" method="POST">
@csrf
<div class="form-group">
<label for="name">Name:</label>
<input type="text" name="name" class="form-control" required>
</div>
<div class="form-group">
<label for="email">Email:</label>
<input type="email" name="email" class="form-control" required>
</div>
<div class="form-group">
<label for="comment">Comment:</label>
<textarea name="comment" class="form-control" rows="5" required></textarea>
</div>
<button type="submit" class="btn btn-primary">Submit</button>
</form>
</div>
</div>
</body>
</html>
Step 5: Defining Routes
Define routes in the routes/web.php
file:
use App\Http\Controllers\CommentController;
Route::get('/', function () {
return view('comment_form');
});
Route::post('/comments', [CommentController::class, 'store'])->name('comments.store');
Step 6: Running the Application
Run your Laravel application:
php artisan serve
Now, visit http://127.0.0.1:8000
in your browser to see the comment form.
This setup will give you a functional and styled comment form in your Laravel application. You can further enhance the design and functionality as needed.