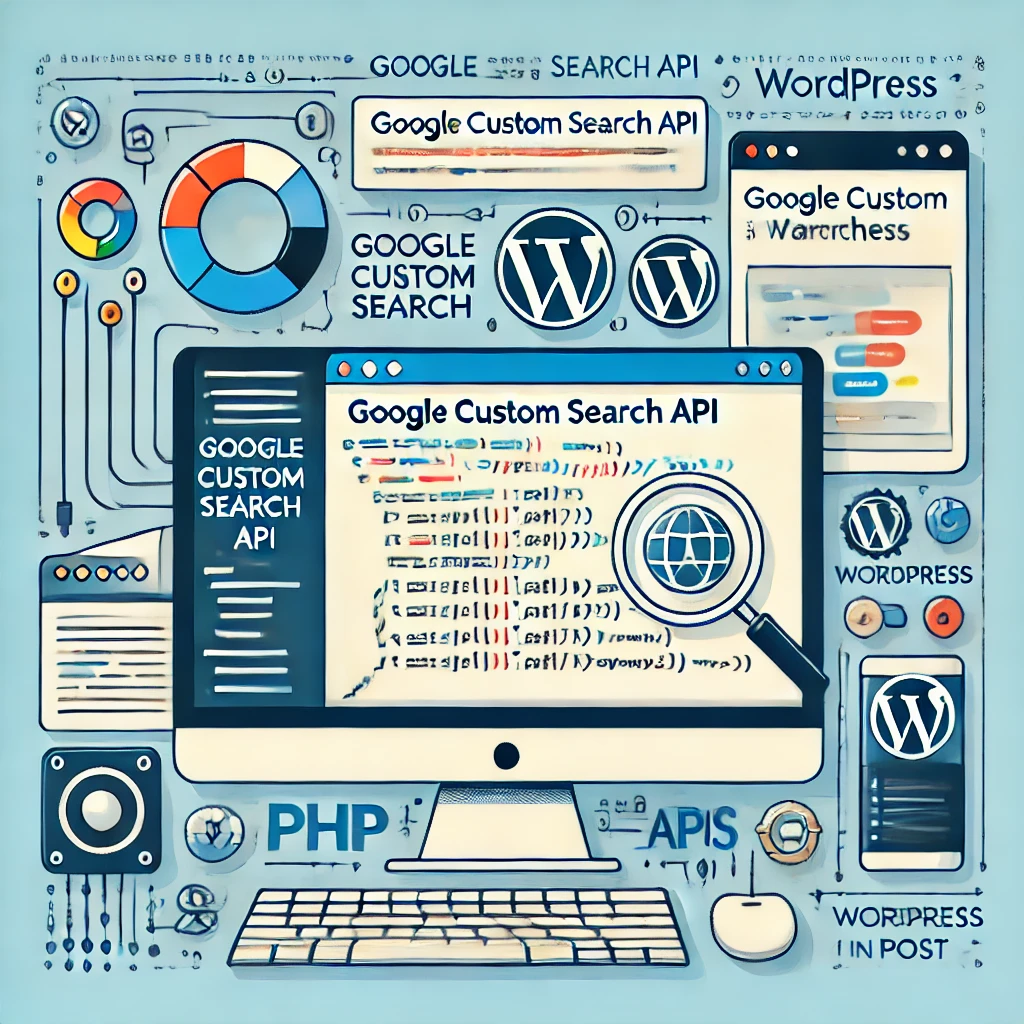
In this blog post, we will explore a PHP script that utilizes the Google Custom Search API to search for images based on user-defined keywords. We’ll also cover how to create a WordPress post from the results. This application demonstrates how to integrate Google’s powerful search capabilities into your own applications, providing an easy way to retrieve and display images related to specific queries.
Code Breakdown
1. Setting Up the Environment
The code begins with defining two main functions: searchImages
and createWordPressPost
. The searchImages
function handles image searches via Google’s Custom Search API, while the createWordPressPost
function is responsible for publishing the search results on a WordPress site.
API Key and Custom Search Engine ID
To use the Google Custom Search API, you must have an API key and a Custom Search Engine (CSE) ID. These credentials are required to authenticate your requests:
$apiKey = 'YOUR_GOOGLE_API_KEY';
$searchEngineId = 'YOUR_CSE_ID';
2. The searchImages
Function
The searchImages
function takes a search query as input and constructs a URL for the API request. It then performs a cURL request to fetch image results:
function searchImages($query) {
// Create the URL for the request
$url = "https://www.googleapis.com/customsearch/v1?q=" . urlencode($query) . "&cx=$searchEngineId&key=$apiKey&searchType=image";
// Initialize cURL session
$curl = curl_init();
// Set cURL options
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
curl_setopt($curl, CURLOPT_HEADER, false);
// Execute cURL session and get the response
$response = curl_exec($curl);
// Close cURL session
curl_close($curl);
// Decode the JSON response
return json_decode($response, true);
}
3. The createWordPressPost
Function
This function takes the post title and content, prepares the data, and sends it to the WordPress REST API to create a new post:
function createWordPressPost($title, $content) {
$wordpressSite = 'YOUR_WORDPRESS_SITE_URL';
$username = 'YOUR_USERNAME';
$password = 'YOUR_APPLICATION_PASSWORD';
// Prepare the API URL
$apiUrl = $wordpressSite . '/wp-json/wp/v2/posts';
// Prepare the post data
$postData = [
'title' => $title,
'content' => $content,
'status' => 'publish'
];
// Set up the request with basic authentication
$curl = curl_init();
curl_setopt($curl, CURLOPT_URL, $apiUrl);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
curl_setopt($curl, CURLOPT_POST, true);
curl_setopt($curl, CURLOPT_POSTFIELDS, json_encode($postData));
curl_setopt($curl, CURLOPT_HTTPHEADER, [
'Content-Type: application/json',
'Authorization: Basic ' . base64_encode($username . ':' . $password)
]);
// Execute the request and capture response
$response = curl_exec($curl);
curl_close($curl);
return json_decode($response, true);
}
4. Collecting Search Results
The script checks if a keyword is provided through a GET request. If so, it generates several queries based on that keyword:
if (isset($_GET['keyword'])) {
$keyword = $_GET['keyword'];
$queries = [
"What is $keyword",
"Why $keyword?",
"How $keyword Works?",
"$keyword Architecture?",
"How to install and configure $keyword?",
"Basic Tutorial of $keyword"
];
$content = ""; // Initialize content variable for WordPress post
// Collect search results
foreach ($queries as $query) {
$results = searchImages($query);
if (!empty($results['items'])) {
$imageUrl = $results['items'][0]['link'];
$imageTitle = htmlspecialchars($results['items'][0]['title']);
$content .= "<h3>$query</h3><img src='$imageUrl' alt='$imageTitle'><br>";
} else {
$content .= "<p>No results found for '$query'.</p>";
}
}
// Create a WordPress post with the collected content
$postTitle = "Image Search Results for '$keyword'";
$response = createWordPressPost($postTitle, $content);
5. The HTML Form
The HTML section provides a simple interface for users to input their search keyword. The results are displayed below the form:
<form action="" method="get">
<input type="text" name="keyword" placeholder="Enter a keyword e.g., Git">
<button type="submit">Generate Queries</button>
</form>
<div class="result-container">
<?php
if (isset($queries)) {
foreach ($queries as $query) {
$results = searchImages($query);
if (!empty($results['items'])) {
echo "<div class='result-item'>";
echo "<h3>" . htmlspecialchars($query) . "</h3>";
echo "<img src='" . $results['items'][0]['link'] . "' alt='" . htmlspecialchars($results['items'][0]['title']) . "'>";
echo "</div>";
} else {
echo "<p>No results found for '$query'.</p>";
}
}
}
?>
</div>
Conclusion
This PHP script effectively utilizes the Google Custom Search API to fetch images based on user queries and post the results to a WordPress site. It demonstrates how to combine different web technologies to create a dynamic application.
To run this script, ensure that you have valid API credentials and a working WordPress installation. Customize the code as needed to fit your project requirements. Happy coding!