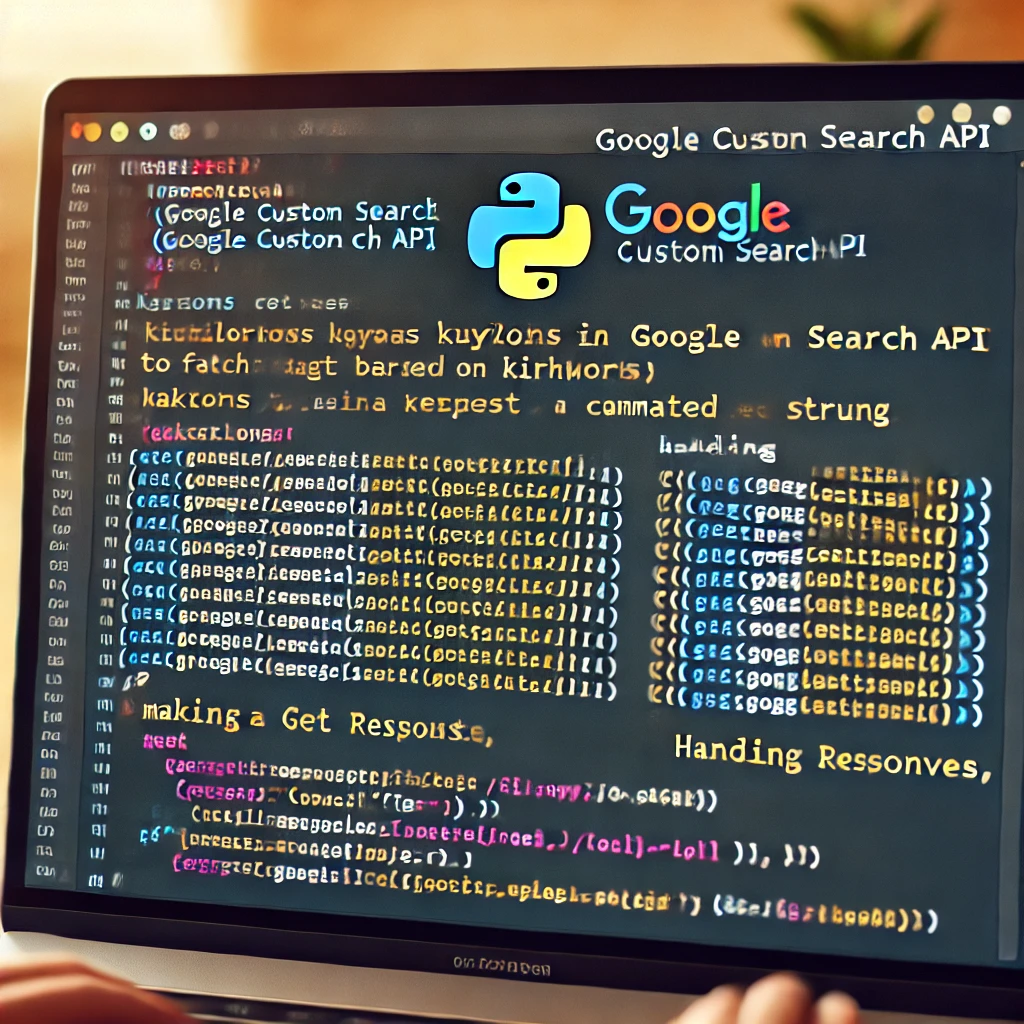
To automatically generate image search queries using Google API, you can use the Custom Search JSON API. Here’s a step-by-step guide:
Step 1: Create a Google Cloud Project and Enable API
- Create a Google Cloud Project on the Google Cloud Console.
- Enable the Custom Search JSON API for your project.
- Create an API Key to authenticate your requests.
Step 2: Set Up a Custom Search Engine (CSE)
- Go to the Custom Search Engine and create a new search engine.
- Specify the sites you want to search or choose to search the entire web (
*.com
). - Note down the Search Engine ID (
cx
), which is required for API calls.
Step 3: Write Code to Generate Image Search Queries
Use the following PHP and HTML code example to generate image search queries using the keywords and fetch images:
<?php
function searchImages($query) {
// Your Google API key and Custom Search Engine ID
$apiKey = 'your_api_key';
$searchEngineId = 'your_search_engine_id';
// Create the URL for the request
$url = "https://www.googleapis.com/customsearch/v1?q=" . urlencode($query) . "&cx=$searchEngineId&key=$apiKey&searchType=image";
// Initialize cURL session
$curl = curl_init();
// Set cURL options
curl_setopt($curl, CURLOPT_URL, $url);
curl_setopt($curl, CURLOPT_RETURNTRANSFER, true);
curl_setopt($curl, CURLOPT_HEADER, false);
// Execute cURL session and get the response
$response = curl_exec($curl);
// Close cURL session
curl_close($curl);
// Decode the JSON response
return json_decode($response, true);
}
// Define search queries based on a user input keyword
if (isset($_GET['keyword'])) {
$keyword = $_GET['keyword'];
$queries = [
"What is $keyword",
"Why $keyword?",
"How $keyword Works?",
"$keyword Architecture?",
"How to install and configure $keyword?",
"Basic Tutorial of $keyword"
];
}
?>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Advanced Image Search</title>
</head>
<body>
<h1>Google Custom Search - Advanced Image Search</h1>
<form action="" method="get">
<input type="text" name="keyword" placeholder="Enter a keyword e.g., Git">
<button type="submit">Generate Queries</button>
</form>
<?php
if (isset($queries)) {
foreach ($queries as $query) {
$results = searchImages($query);
if (!empty($results['items'])) {
echo "<div style='margin-top: 20px;'>";
echo "<h3>" . htmlspecialchars($query) . "</h3>";
echo "<img src='" . $results['items'][0]['link'] . "' alt='" . htmlspecialchars($results['items'][0]['title']) . "' style='width: 200px; height: auto;'><br>";
echo "</div>";
} else {
echo "<p>No results found for '$query'.</p>";
}
}
}
?>
</body>
</html>
How It Works:
- Form Input: Users enter a keyword (e.g., “Git”), which is used to generate a list of search queries.
- Query Execution: For each generated query, the
searchImages
function is called to perform the search and return results. - Results Display: For each query, the first image result is displayed under the query title.
Usage Tips:
- Replace
'your_api_key'
and'your_search_engine_id'
with your actual API key and search engine ID. - To ensure robustness and security in a production environment, consider adding additional error handling, such as checking for cURL errors, and possibly rate limiting or caching responses to enhance performance and reduce the number of API calls.