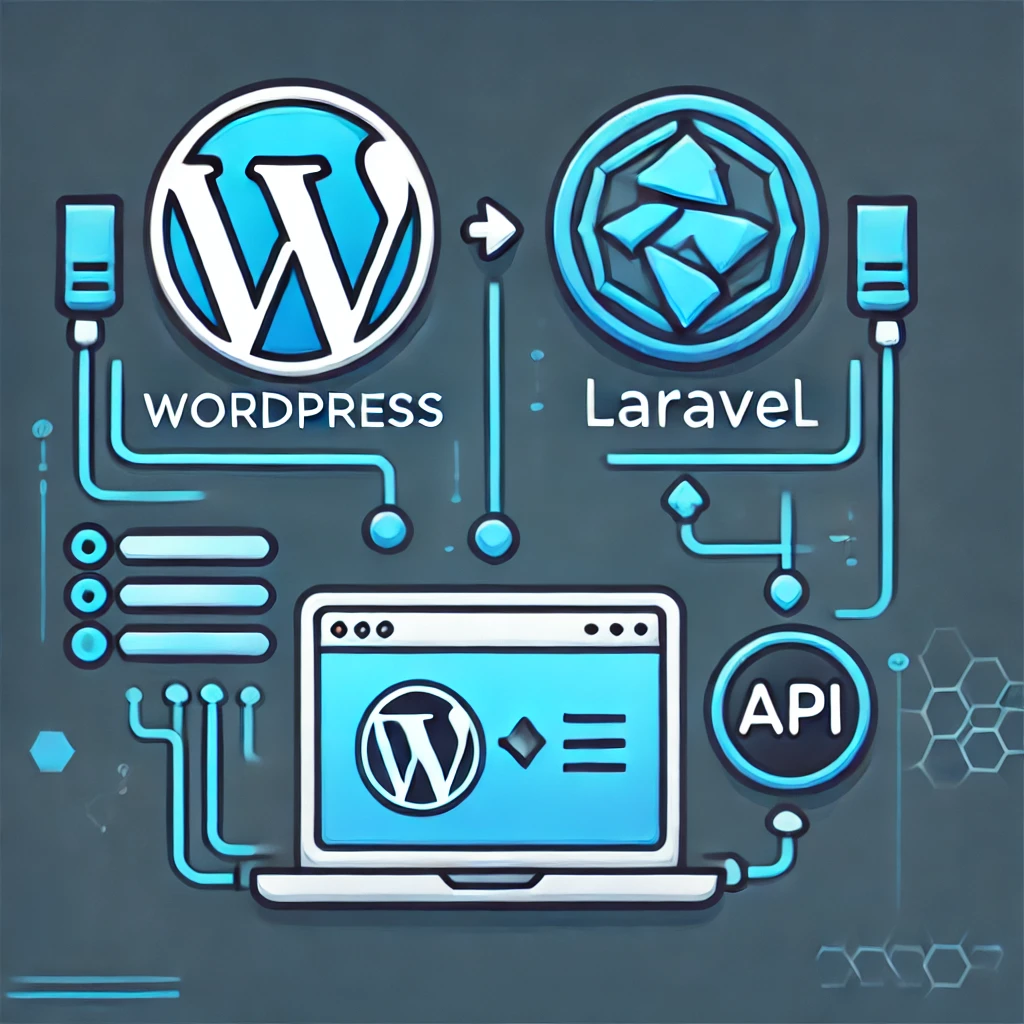
To display recent WordPress posts in a Laravel page, you’ll need to interact with the WordPress database or use the WordPress REST API. Here’s a simple approach using the WordPress REST API to fetch the latest posts and display them on your Laravel page.
Step 1: Fetch WordPress Posts Using REST API
First, WordPress offers a REST API that you can use to fetch recent posts. The URL to get the latest posts is typically:
https://your-wordpress-site.com/wp-json/wp/v2/posts
You can use Laravel’s HTTP client to fetch data from this API.
Step 2: Make an HTTP Request in Laravel
- Install Guzzle (Laravel 8+ uses Guzzle by default, but if you’re on an older version, you may need to install it):
composer require guzzlehttp/guzzle
- Create a Controller Method to Fetch Posts: Inside your Laravel controller, add a method to fetch and display the WordPress posts:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Http;
class BlogController extends Controller
{
public function showRecentPosts()
{
// Fetch the latest posts from the WordPress REST API
$response = Http::get('https://your-wordpress-site.com/wp-json/wp/v2/posts');
// Check if the request was successful
if ($response->successful()) {
// Get the posts data
$posts = $response->json();
} else {
$posts = [];
}
// Pass the posts data to a view
return view('blog.recent-posts', compact('posts'));
}
}
Step 3: Create a View to Display the Posts
Create a view (e.g., resources/views/blog/recent-posts.blade.php
) to display the posts:
@extends('layouts.app')
@section('content')
<div class="container">
<h1>Recent WordPress Posts</h1>
@if(!empty($posts))
@foreach($posts as $post)
<div class="post">
<h2>{{ $post['title']['rendered'] }}</h2>
<div>{!! $post['excerpt']['rendered'] !!}</div>
<a href="{{ $post['link'] }}" target="_blank">Read more</a>
</div>
<hr>
@endforeach
@else
<p>No posts available.</p>
@endif
</div>
@endsection
Step 4: Define a Route for Displaying Posts
In your routes/web.php
file, define a route for this:
use App\Http\Controllers\BlogController;
Route::get('/recent-posts', [BlogController::class, 'showRecentPosts']);
Step 5: Test the Page
Now, when you visit /recent-posts
in your Laravel application, it should display the recent posts from your WordPress site.
Customization
You can customize the way the posts are fetched, including limiting the number of posts by adding query parameters to the API request:
https://your-wordpress-site.com/wp-json/wp/v2/posts?per_page=5
This will limit the results to the 5 most recent posts.
That’s it! You now have a Laravel page showing recent WordPress posts using the REST API.