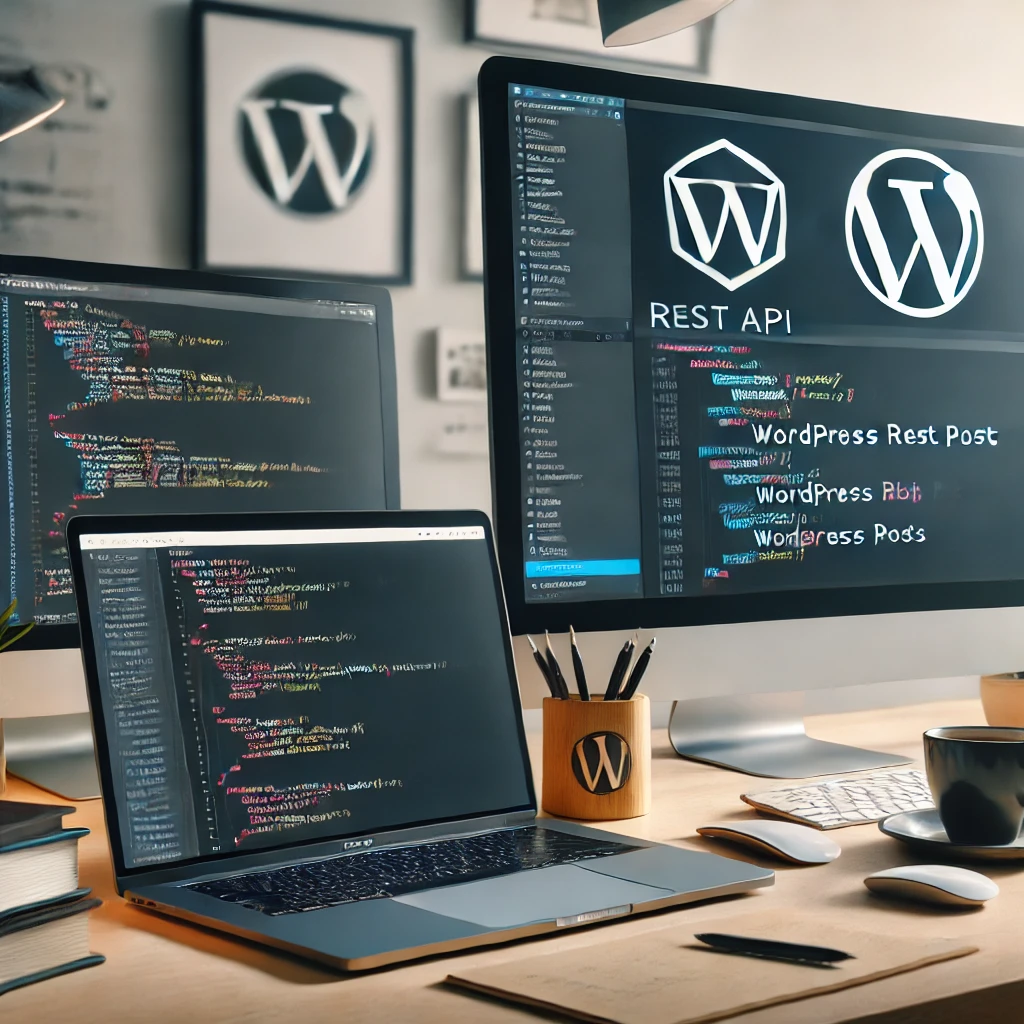
To run the Laravel page and view the recent WordPress posts, you’ll need to follow these steps. This assumes you already have a Laravel project set up. If not, I’ll also guide you through setting it up.
Step 1: Ensure Laravel Is Installed
If you don’t have a Laravel project set up, you can create one using Composer:
composer create-project --prefer-dist laravel/laravel my-laravel-app
After that, navigate to the project folder:
cd my-laravel-app
Step 2: Create the Controller
Run the Artisan command to create the controller if you haven’t already done so:
php artisan make:controller BlogController
Then, open the newly created controller at app/Http/Controllers/BlogController.php
and add the method to fetch the WordPress posts as described earlier:
<?php
namespace App\Http\Controllers;
use Illuminate\Support\Facades\Http;
class BlogController extends Controller
{
public function showRecentPosts()
{
// Fetch the latest posts from the WordPress REST API
$response = Http::get('https://your-wordpress-site.com/wp-json/wp/v2/posts');
if ($response->successful()) {
$posts = $response->json();
} else {
$posts = [];
}
return view('blog.recent-posts', compact('posts'));
}
}
Step 3: Create the View
Next, create a Blade view to display the recent posts. In your project directory, go to resources/views/blog
and create a file called recent-posts.blade.php
. Add the following content:
@extends('layouts.app')
@section('content')
<div class="container">
<h1>Recent WordPress Posts</h1>
@if(!empty($posts))
@foreach($posts as $post)
<div class="post">
<h2>{{ $post['title']['rendered'] }}</h2>
<div>{!! $post['excerpt']['rendered'] !!}</div>
<a href="{{ $post['link'] }}" target="_blank">Read more</a>
</div>
<hr>
@endforeach
@else
<p>No posts available.</p>
@endif
</div>
@endsection
If you don’t have a layout already, you can create a basic one at resources/views/layouts/app.blade.php
:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Laravel Blog</title>
<link rel="stylesheet" href="{{ asset('css/app.css') }}">
</head>
<body>
<nav>
<a href="/">Home</a>
<a href="/recent-posts">Recent Posts</a>
</nav>
<main>
@yield('content')
</main>
<script src="{{ asset('js/app.js') }}"></script>
</body>
</html>
Step 4: Set Up Routing
In your routes/web.php
file, add a route for the page:
use App\Http\Controllers\BlogController;
Route::get('/recent-posts', [BlogController::class, 'showRecentPosts']);
Step 5: Run the Laravel Development Server
Now you need to run the Laravel development server. Use this command to start the server:
php artisan serve
This will start the development server, typically at http://127.0.0.1:8000
.
Step 6: Access the Page
Once the server is running, open your browser and visit the following URL to see your recent WordPress posts:
http://127.0.0.1:8000/recent-posts
If everything is set up correctly, this page should display the recent posts from your WordPress site using the REST API.
Troubleshooting
- Permissions: Ensure that the WordPress REST API is enabled and accessible. You can check by visiting
https://your-wordpress-site.com/wp-json/wp/v2/posts
in your browser. - Dependencies: If the
Http
class isn’t available, you may need to add it to the service providers (if you are on a Laravel version that doesn’t include it by default). - CSS/JS assets: If you want to style the page, you can add CSS and JS files in the
public/css
andpublic/js
folders respectively, and link them in theapp.blade.php
layout.
That’s how you can run this page and view recent WordPress posts in your Laravel application.