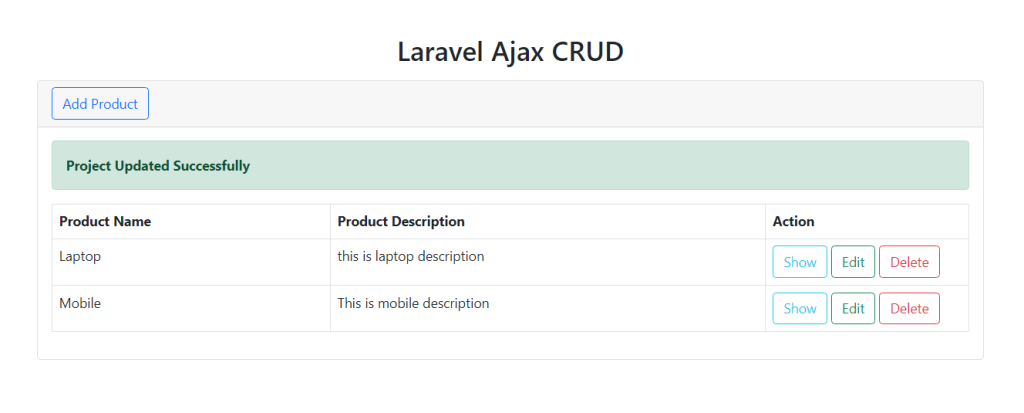
Introduction:
In this blog, you’ll learn how to develop a Laravel 11 AJAX CRUD application through an easy step-by-step tutorial. But before we dive in, let’s start with some introductions:
Laravel is a free and open-source PHP web framework designed for building web applications using the MVC (Model-View-Controller) architectural pattern. It simplifies web development by providing built-in features that speed up and ease the process.
AJAX (Asynchronous JavaScript and XML) is a collection of web development techniques that use various web technologies to enable web applications to operate asynchronously, enhancing user experience.
CRUD stands for Create, Read, Update, and Delete—the four fundamental operations in any programming environment, typically related to database management:
- Create: Generate new records.
- Read: Retrieve or view existing records.
- Update: Modify existing records.
- Delete: Remove or destroy records.
Prerequisite:
Composer
MySQL
PHP >= 8.2
Step 1: Install Laravel 11
First, choose a folder where you want to install Laravel. Then, run the following command in Terminal or CMD to install Laravel 11:
Install via Composer:
composer create-project laravel/laravel ajax-crud
Step 2: Configure Database Settings
Once the installation is complete, open the .env
file and configure the database settings as follows:
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=your_database_name (e.g., crud_ajax)
DB_USERNAME=your_database_username (e.g., root)
DB_PASSWORD=your_database_password (e.g., root)
Step 3: Create Model and Database Migration
- Model: A class that represents a database table.
- Migration: Functions like version control for the database, enabling you to modify and share the database schema with your team.
Run the following command in the Terminal or CMD to create both a model and a migration:
php artisan make:model Project --migration
After executing this command, you’ll find a new file in the database/migrations
directory. Add the code below to this migration file.
<?php
use Illuminate\Database\Migrations\Migration;
use Illuminate\Database\Schema\Blueprint;
use Illuminate\Support\Facades\Schema;
return new class extends Migration
{
/**
* Run the migrations.
*/
public function up(): void
{
Schema::create('projects', function (Blueprint $table) {
$table->id();
$table->string('name');
$table->text('description');
$table->timestamps();
});
}
/**
* Reverse the migrations.
*/
public function down(): void
{
Schema::dropIfExists('projects');
}
};
Execute the migration by running the following Artisan command:
php artisan migrate
Step 4: Create a Resource Controller
A Resource Controller manages all HTTP requests related to a specific resource within your application.
To generate a Resource Controller, run the following command:
php artisan make:controller ProjectController --api
This command will create a new controller file at app/Http/Controllers/ProjectController.php
. The file will include methods for each of the standard resource operations. Open this file and add the necessary code as outlined below:
app/Http/Controllers/ProjectController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Project;
class ProjectController extends Controller
{
/**
* Display a listing of the resource.
*/
public function index()
{
$projects = Project::get();
return response()->json(['projects' => $projects]);
}
/**
* Store a newly created resource in storage.
*/
public function store(Request $request)
{
request()->validate([
'name' => 'required|max:255',
'description' => 'required',
]);
$project = new Project();
$project->name = $request->name;
$project->description = $request->description;
$project->save();
return response()->json(['status' => "success"]);
}
/**
* Display the specified resource.
*/
public function show(string $id)
{
$project = Project::find($id);
return response()->json(['project' => $project]);
}
/**
* Update the specified resource in storage.
*/
public function update(Request $request, string $id)
{
request()->validate([
'name' => 'required|max:255',
'description' => 'required',
]);
$project = Project::find($id);
$project->name = $request->name;
$project->description = $request->description;
$project->save();
return response()->json(['status' => "success"]);
}
/**
* Remove the specified resource from storage.
*/
public function destroy(string $id)
{
Project::destroy($id);
return response()->json(['status' => "success"]);
}
}
Step 5: Register Routes
Once you have created the resource controller, you need to register both the resourceful route and the view route.
In routes/web.php
:
<?php
use Illuminate\Support\Facades\Route;
use App\Http\Controllers\ProjectController;
Route::get('/', function () {
return view('projects');
});
Route::apiResource('projects', ProjectController::class);
Step 6: Create a Blade File
Blade is Laravel’s simple yet powerful templating engine. Blade view files have the .blade.php
extension and are typically stored in the resources/views/
directory.
To enhance the appearance of our simple CRUD app, we will use Bootstrap. Bootstrap is a popular, free, open-source CSS framework designed for building responsive and mobile-first web projects.
Create a Blade file named projects.blade.php
in the resources/views/
directory. After creating the file, insert the following code:
resources/views/projects.blade.php
<!DOCTYPE html>
<html lang="en">
<head>
<title>Laravel Ajax CRUD</title>
<meta charset="utf-8">
<meta name="csrf-token" content="{{ csrf_token() }}">
<meta name="app-url" content="{{ url('/') }}">
<meta name="viewport" content="width=device-width, initial-scale=1">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.4.1/jquery.min.js"></script>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js" integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM" crossorigin="anonymous"></script>
</head>
<body>
<div class="container">
<h2 class="text-center mt-5 mb-3">Laravel Ajax CRUD</h2>
<div class="card">
<div class="card-header">
<button class="btn btn-outline-primary" onclick="createProject()">
Add Product
</button>
</div>
<div class="card-body">
<div id="alert-div">
</div>
<table class="table table-bordered">
<thead>
<tr>
<th>Product Name</th>
<th>Product Description</th>
<th width="240px">Action</th>
</tr>
</thead>
<tbody id="projects-table-body">
</tbody>
</table>
</div>
</div>
</div>
<!-- modal for creating and editing function -->
<div class="modal" tabindex="-1" id="form-modal">
<div class="modal-dialog" >
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title">Product Form</h5>
<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>
</div>
<div class="modal-body">
<div id="error-div"></div>
<form>
<input type="hidden" name="update_id" id="update_id">
<div class="form-group">
<label for="name">Product Name</label>
<input type="text" class="form-control" id="name" name="name">
</div>
<div class="form-group">
<label for="description">Product Description</label>
<textarea class="form-control" id="description" rows="3" name="description"></textarea>
</div>
<button type="submit" class="btn btn-outline-primary mt-3" id="save-project-btn">Save Product</button>
</form>
</div>
</div>
</div>
</div>
<!-- view record modal -->
<div class="modal" tabindex="-1" id="view-modal">
<div class="modal-dialog" >
<div class="modal-content">
<div class="modal-header">
<h5 class="modal-title">Project Information</h5>
<button type="button" class="btn-close" data-bs-dismiss="modal" aria-label="Close"></button>
</div>
<div class="modal-body">
<b>Name:</b>
<p id="name-info"></p>
<b>Description:</b>
<p id="description-info"></p>
</div>
</div>
</div>
</div>
<script type="text/javascript">
showAllProjects();
/*
This function will get all the project records
*/
function showAllProjects()
{
let url = $('meta[name=app-url]').attr("content") + "/projects";
$.ajax({
url: url,
type: "GET",
success: function(response) {
$("#projects-table-body").html("");
let projects = response.projects;
for (var i = 0; i < projects.length; i++)
{
let showBtn = '<button ' +
' class="btn btn-outline-info" ' +
' onclick="showProject(' + projects[i].id + ')">Show' +
'</button> ';
let editBtn = '<button ' +
' class="btn btn-outline-success" ' +
' onclick="editProject(' + projects[i].id + ')">Edit' +
'</button> ';
let deleteBtn = '<button ' +
' class="btn btn-outline-danger" ' +
' onclick="destroyProject(' + projects[i].id + ')">Delete' +
'</button>';
let projectRow = '<tr>' +
'<td>' + projects[i].name + '</td>' +
'<td>' + projects[i].description + '</td>' +
'<td>' + showBtn + editBtn + deleteBtn + '</td>' +
'</tr>';
$("#projects-table-body").append(projectRow);
}
},
error: function(response) {
console.log(response.responseJSON)
}
});
}
/*
check if form submitted is for creating or updating
*/
$("#save-project-btn").click(function(event ){
event.preventDefault();
if($("#update_id").val() == null || $("#update_id").val() == "")
{
storeProject();
} else {
updateProject();
}
})
/*
show modal for creating a record and
empty the values of form and remove existing alerts
*/
function createProject()
{
$("#alert-div").html("");
$("#error-div").html("");
$("#update_id").val("");
$("#name").val("");
$("#description").val("");
$("#form-modal").modal('show');
}
/*
submit the form and will be stored to the database
*/
function storeProject()
{
$("#save-project-btn").prop('disabled', true);
let url = $('meta[name=app-url]').attr("content") + "/projects";
let data = {
name: $("#name").val(),
description: $("#description").val(),
};
$.ajax({
headers: {
'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content')
},
url: url,
type: "POST",
data: data,
success: function(response) {
$("#save-project-btn").prop('disabled', false);
let successHtml = '<div class="alert alert-success" role="alert"><b>Project Created Successfully</b></div>';
$("#alert-div").html(successHtml);
$("#name").val("");
$("#description").val("");
showAllProjects();
$("#form-modal").modal('hide');
},
error: function(response) {
$("#save-project-btn").prop('disabled', false);
/*
show validation error
*/
if (typeof response.responseJSON.errors !== 'undefined')
{
let errors = response.responseJSON.errors;
let descriptionValidation = "";
if (typeof errors.description !== 'undefined')
{
descriptionValidation = '<li>' + errors.description[0] + '</li>';
}
let nameValidation = "";
if (typeof errors.name !== 'undefined')
{
nameValidation = '<li>' + errors.name[0] + '</li>';
}
let errorHtml = '<div class="alert alert-danger" role="alert">' +
'<b>Validation Error!</b>' +
'<ul>' + nameValidation + descriptionValidation + '</ul>' +
'</div>';
$("#error-div").html(errorHtml);
}
}
});
}
/*
edit record function
it will get the existing value and show the Product Form
*/
function editProject(id)
{
let url = $('meta[name=app-url]').attr("content") + "/projects/" + id ;
$.ajax({
url: url,
type: "GET",
success: function(response) {
let project = response.project;
$("#alert-div").html("");
$("#error-div").html("");
$("#update_id").val(project.id);
$("#name").val(project.name);
$("#description").val(project.description);
$("#form-modal").modal('show');
},
error: function(response) {
console.log(response.responseJSON)
}
});
}
/*
sumbit the form and will update a record
*/
function updateProject()
{
$("#save-project-btn").prop('disabled', true);
let url = $('meta[name=app-url]').attr("content") + "/projects/" + $("#update_id").val();
let data = {
id: $("#update_id").val(),
name: $("#name").val(),
description: $("#description").val(),
};
$.ajax({
headers: {
'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content')
},
url: url,
type: "PUT",
data: data,
success: function(response) {
$("#save-project-btn").prop('disabled', false);
let successHtml = '<div class="alert alert-success" role="alert"><b>Project Updated Successfully</b></div>';
$("#alert-div").html(successHtml);
$("#name").val("");
$("#description").val("");
showAllProjects();
$("#form-modal").modal('hide');
},
error: function(response) {
/*
show validation error
*/
$("#save-project-btn").prop('disabled', false);
if (typeof response.responseJSON.errors !== 'undefined')
{
console.log(response)
let errors = response.responseJSON.errors;
let descriptionValidation = "";
if (typeof errors.description !== 'undefined')
{
descriptionValidation = '<li>' + errors.description[0] + '</li>';
}
let nameValidation = "";
if (typeof errors.name !== 'undefined')
{
nameValidation = '<li>' + errors.name[0] + '</li>';
}
let errorHtml = '<div class="alert alert-danger" role="alert">' +
'<b>Validation Error!</b>' +
'<ul>' + nameValidation + descriptionValidation + '</ul>' +
'</div>';
$("#error-div").html(errorHtml);
}
}
});
}
/*
get and display the record info on modal
*/
function showProject(id)
{
$("#name-info").html("");
$("#description-info").html("");
let url = $('meta[name=app-url]').attr("content") + "/projects/" + id +"";
$.ajax({
url: url,
type: "GET",
success: function(response) {
let project = response.project;
$("#name-info").html(project.name);
$("#description-info").html(project.description);
$("#view-modal").modal('show');
},
error: function(response) {
console.log(response.responseJSON)
}
});
}
/*
delete record function
*/
function destroyProject(id)
{
let url = $('meta[name=app-url]').attr("content") + "/projects/" + id;
let data = {
name: $("#name").val(),
description: $("#description").val(),
};
$.ajax({
headers: {
'X-CSRF-TOKEN': $('meta[name="csrf-token"]').attr('content')
},
url: url,
type: "DELETE",
data: data,
success: function(response) {
let successHtml = '<div class="alert alert-success" role="alert"><b>Project Deleted Successfully</b></div>';
$("#alert-div").html(successHtml);
showAllProjects();
},
error: function(response) {
console.log(response.responseJSON)
}
});
}
</script>
</body>
</html>
Step 7: Run the Application
Once you have completed the previous steps, start your application by executing the following command:
php artisan serve
After the application is running, open your browser and navigate to:
http://localhost:8000
Screenshots:
Laravel 11 AJAX CRUD Application – Product Index Page:
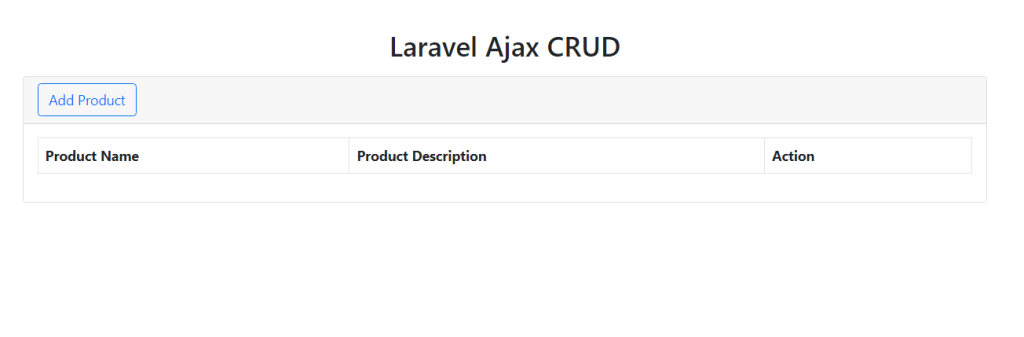
Laravel 11 AJAX CRUD Application: Product Creation Form Modal
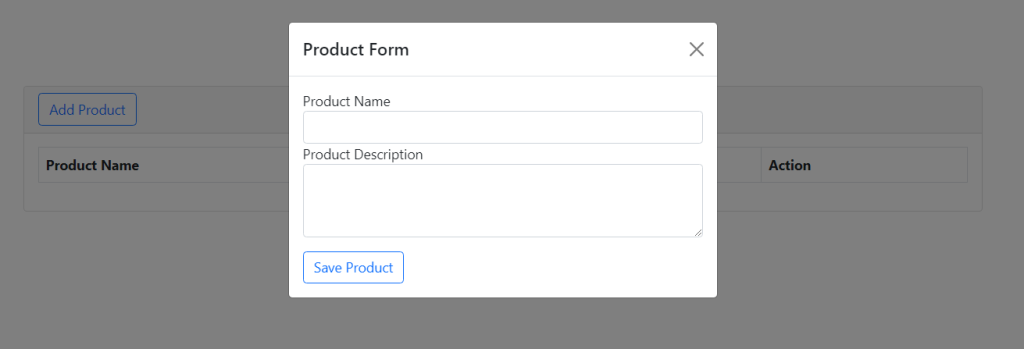
Laravel 11 AJAX CRUD App (edit product form modal):
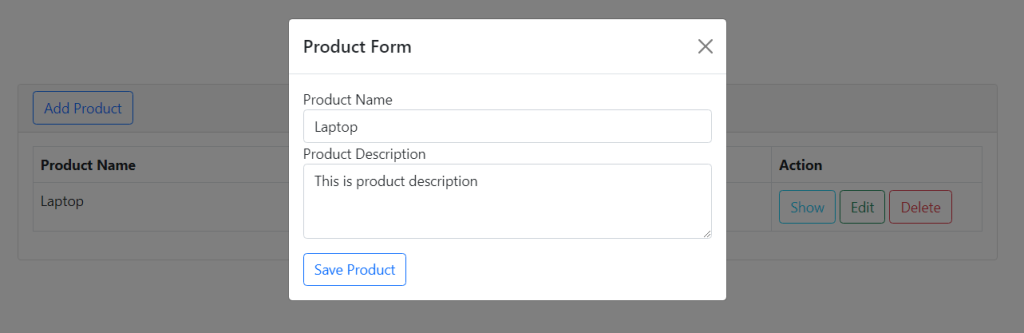
Laravel 11 AJAX CRUD Application: Show Product Information in a Modal
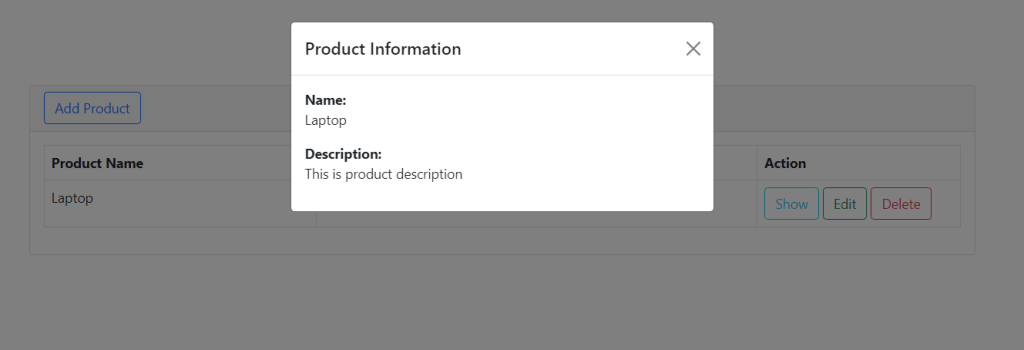
Thank you for following along with this blog post series! I hope you found the step-by-step guides and explanations helpful in building and understanding your Laravel AJAX CRUD application. Your dedication to learning and exploring new concepts is truly commendable. If you have any questions or need further assistance, please feel free to reach out. Keep coding and continue enhancing your skills. Happy developing!