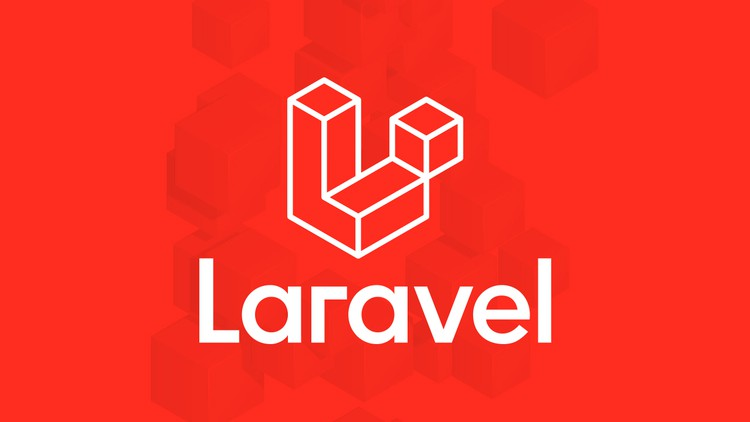
What is Laravel ?
Laravel is a popular PHP web application framework known for its elegant syntax and robust features that make web development faster and easier. It follows the Model-View-Controller (MVC) architectural pattern, which organizes the application structure into three main areas: data management (Model), user interface (View), and data processing (Controller).
Key Features of Laravel:
- Eloquent ORM (Object-Relational Mapping): Laravel includes a built-in ORM system that provides a simple ActiveRecord implementation for working with your database. This allows developers to interact with databases in a more intuitive and streamlined way.
- Routing: Laravel provides a simple, expressive way to define routes by using a straightforward closure or controller-based approach. Routes are defined in the web routes file and can determine how data is transferred between views and controllers.
- Middleware: These are layers that can filter HTTP requests entering your application. For example, Laravel includes a middleware for verifying a user’s authentication and if the user is not authenticated, it redirects them to the login screen.
- Blade Templating Engine: This is Laravel’s powerful templating engine which allows data to be easily displayed in views without cluttering PHP code with HTML. Blade templates are compiled into plain PHP and cached until they are modified, meaning they are not recompiled on each request.
- Security: Laravel provides robust security features that are very easy to implement. It guards against various risks like SQL injection, cross-site request forgery, and cross-site scripting. Laravel uses hashed and salted password schemes so the password would never be saved as plain text in the database.
- Artisan Console: Laravel includes a built-in tool for command-line called Artisan which helps automate repetitive and tedious programming tasks. These Artisan commands can also be extended to perform custom tasks.
- Testing: Laravel is built with testing in mind. Integration with PHP Unit is out-of-the-box, and it provides helpful methods for simulating basic behaviors.
- Task Scheduling: Task scheduling in Laravel offers a fluent, expressive way to define command schedule within the framework, only requiring a single Cron entry on the server.
- Events and Broadcasting: Laravel allows easy implementation of events, listeners, and event broadcasting. This feature is useful for implementing real-time data updates and web sockets.
- Package Development: Laravel supports multiple ways of developing packages, allowing you to package application code and re-use it in other Laravel projects.
Why we use Laravel?
Laravel is highly regarded for its ease of use, scalability, and a variety of features that make it a go-to framework for many developers. Here’s a detailed breakdown of the points you mentioned:
- Popular: Laravel is one of the most popular PHP frameworks due to its comprehensive features, community support, and continuous improvements. Its popularity also means a wealth of tutorials, forums, and third-party packages that enhance its functionality and ease of use.
- Get Rid from Blocks: Laravel helps streamline coding workflow by removing unnecessary repetitive coding tasks. This can refer to its elegant syntax and the use of tools like Eloquent ORM and Blade templating which simplify complex coding tasks.
- Easy to Use: Laravel’s intuitive interface and workflow make it accessible to newcomers while providing powerful features for experienced developers. Its extensive documentation and tutorials further ease the learning curve.
- Tons of Predefined Functions: Laravel comes with numerous built-in functionalities, such as user authentication, sessions, and routing, which help developers to avoid writing boilerplate code and focus on application logic.
- Code Reusability: Laravel’s use of packages and service providers allows for easy reusability of code. Developers can package functionality and re-use it across multiple projects, or even share it with the community.
- Secured: Laravel takes security seriously and offers various ways to protect applications from common threats like SQL injection, cross-site request forgery (CSRF), and cross-site scripting (XSS). It manages security within the framework itself, ensuring that database and data handling practices are as secure as possible.
- Improved Coding Experience: With tools like Artisan, Laravel’s command line interface, developers can automate repetitive tasks, enhance performance, and manage database migrations efficiently. The MVC architecture helps maintain clean and manageable code.
- Complex Web Application: Laravel is robust enough to handle complex web applications. It provides a strong foundation with its built-in features, extensive community packages, and the ability to integrate with modern front-end frameworks.
- API Creation: Laravel is excellent for API development, thanks to its built-in support for data transformation layers, authentication tokens, and its ability to handle RESTful routing smoothly.
What pre-requisite knowledge you required?
To effectively use Laravel, having a foundation in several key areas will set you up for success. Here’s a breakdown of the pre-requisite knowledge you mentioned, along with why each is important:
- PHP: Since Laravel is a PHP framework, a solid understanding of PHP is crucial. This includes knowledge of PHP syntax, functions, and core concepts.
- PHP (OOPs): Laravel makes extensive use of PHP’s object-oriented programming features, including classes, inheritance, and interfaces. Understanding OOP principles in PHP is essential for using Laravel effectively, as it’s built on these concepts.
- MySQL: Laravel frequently interacts with databases, and MySQL is one of the most common database systems used. Knowledge of creating databases, writing queries, and managing data is important because Laravel uses Eloquent ORM to interact with the database using an object-oriented approach.
- MVC Pattern: Laravel is based on the Model-View-Controller (MVC) architectural pattern. Understanding how MVC works (separating logic, UI, and data handling) is important for organizing code in Laravel. This helps in maintaining a clean and modular codebase.
- Command Line (CMD) Basics: Laravel uses a command-line tool called Artisan for various tasks such as migration, job queues, and running tests. Basic command line skills are necessary to navigate and execute these tasks efficiently.
In addition to these, familiarity with the following can also be very helpful:
- Composer: Knowledge of Composer, a dependency manager for PHP, is useful for managing Laravel installations and package dependencies.
- Version Control (e.g., Git): Basic understanding of version control, particularly Git, is advantageous for managing changes and collaborating in projects.
- Front-end Technologies: While not strictly necessary, knowing basic HTML, CSS, and JavaScript can be beneficial, especially if you’re working on full-stack applications. Familiarity with front-end frameworks like Vue.js or React can also be advantageous since Laravel pairs well with these technologies.
Starting with a strong base in these areas will make your Laravel learning journey smoother and more effective.
Requirements for laravel?
To set up a Laravel development environment, there are specific requirements and tools you’ll need to install. Here’s a detailed list based on the essentials you mentioned:
1. Server Requirements
Laravel has a few system requirements. You must ensure your server meets the following requirements:
- PHP Version: Laravel requires PHP 8.0 or higher.
- PHP Extensions: Certain PHP extensions are required, including:
- BCMath PHP Extension
- Ctype PHP Extension
- Fileinfo PHP extension
- JSON PHP Extension
- Mbstring PHP Extension
- OpenSSL PHP Extension
- PDO PHP Extension
- Tokenizer PHP Extension
- XML PHP Extension
2. Web Server
While Laravel does not specifically require Apache or Nginx, it can run on any web server that supports PHP. XAMPP provides an easy package for this because it includes Apache along with MySQL, PHP, and Perl.
- XAMPP: Using XAMPP 8 or later versions is a good choice as it aligns with Laravel’s PHP version requirement. XAMPP simplifies the process of configuring PHP, MySQL, and Apache on your local machine.
3. Composer
- Composer: Laravel uses Composer to manage its dependencies. Therefore, before creating a Laravel project, you need to install Composer. This tool helps you manage libraries that your project requires.
4. Database
- MySQL: As you mentioned using XAMPP, MySQL comes bundled with it, which Laravel can utilize for database operations. Laravel supports several database systems including MySQL, PostgreSQL, SQLite, and SQL Server.
5. Node.js and NPM (Optional)
- Node.js and NPM: While not strictly required, installing Node.js and npm can be beneficial if you plan to use Laravel Mix or any other Node.js based packages or tools for your front-end assets.
6. IDE or Text Editor
- Integrated Development Environment (IDE) or Text Editor: While Laravel does not require a specific IDE or text editor, using one like PhpStorm, Visual Studio Code, or Sublime Text can greatly enhance your coding experience. These often have features such as syntax highlighting, code completion, and more, tailored to Laravel development.
7. Git (Optional)
- Version Control: Using Git for version control is recommended, especially if you plan on collaborating with others or maintaining different versions and branches of your project.
Setup Process
- Install XAMPP: Install XAMPP to set up a local server environment.
- Install Composer: Download and install Composer from its official website.
- Create a Laravel Project: Use Composer to create a new Laravel project by running
composer create-project --prefer-dist laravel/laravel projectName
. - Start Development Server: Use Laravel’s built-in development server by navigating to your project directory in the command line and running
php artisan serve
. This will make your application accessible atlocalhost:8000
by default.
With these requirements met and tools installed, you’ll be ready to start developing with Laravel in a local environment efficiently.
Choosing the Right Laravel Version
When deciding which version of Laravel to learn, it’s usually best to focus on the most recent stable version. As of mid-2024, Laravel 9 is the latest stable release, and it would be advisable to start learning with this version for several reasons:
- Long-Term Support: Laravel 9 is an LTS (Long-Term Support) release, which means it will receive bug fixes until August 2025 and security fixes until August 2026. This extended support period makes it an ideal choice for both learning and production environments.
- Latest Features: Learning the most current version allows you to take advantage of the latest features, improvements, and best practices in Laravel development. New releases often include enhancements in performance, security, and developer tools that can significantly improve development efficiency and application quality.
- Community and Resources: As the Laravel community moves forward, more tutorials, guides, and third-party packages will focus on supporting the latest versions. This means you’ll find more up-to-date resources and community support for Laravel 9.
- Compatibility with PHP: Laravel 9 requires PHP 8.0 or higher, aligning with the ongoing support and security updates for PHP. Learning Laravel 9 ensures that you are also up-to-date with the latest PHP features and improvements, such as named arguments, attributes, and match expressions.
- Preparation for Future Versions: Starting with Laravel 9 prepares you for future updates and versions since understanding the core concepts and latest functionalities will make it easier to adapt to subsequent releases.
By starting with Laravel 9, you ensure that your skills are modern and relevant, and you position yourself well within the ecosystem for professional development or personal projects.