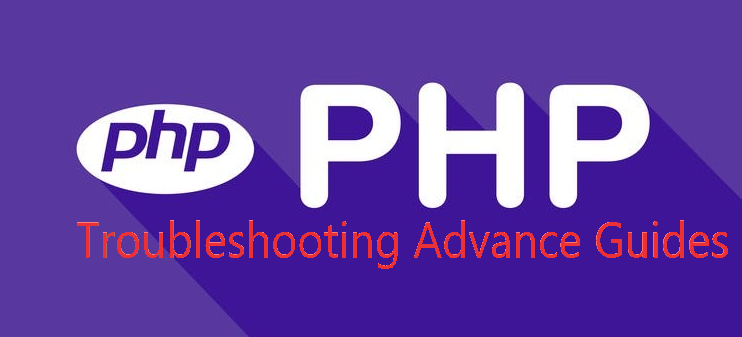
Troubleshooting PHP can be challenging, especially with advanced issues. Here are some advanced guides and tips for diagnosing and resolving PHP problems:
1. Enable Detailed Error Reporting
Ensure PHP is configured to display detailed errors. This can help identify issues more quickly.
ini_set('display_errors', 1);
ini_set('display_startup_errors', 1);
error_reporting(E_ALL);
2. Use PHP’s Built-In Functions
PHP offers several functions for debugging:
error_log()
: Log an error message.debug_backtrace()
: Generate a backtrace.var_dump()
,print_r()
,var_export()
: Display structured information about variables.
3. Check PHP Error Logs
PHP errors are often logged in server logs. Check the error_log
directive in your php.ini
to locate the log file.
4. Analyze Core Dumps
For crashes, generate and analyze core dumps. This requires configuring PHP with --enable-debug
and setting up core dump generation on your server.
5. Use a Debugger
Xdebug is a popular tool for debugging PHP applications. It allows for step-by-step execution and inspection of code.
Installation:
pecl install xdebug
Configuration:
Add the following to your php.ini
:
zend_extension=xdebug.so
xdebug.remote_enable=1
xdebug.remote_host=127.0.0.1
xdebug.remote_port=9000
6. Profiling PHP Code
Profiling can identify performance bottlenecks.
Xdebug Profiling:
xdebug.profiler_enable=1
xdebug.profiler_output_dir=/path/to/profiles
Analyze the profiles with tools like KCachegrind.
7. Monitor Performance
Use tools like New Relic, Blackfire, or Tideways for performance monitoring and profiling.
8. Check for Memory Leaks
Use tools like Valgrind or built-in PHP functions to monitor memory usage.
Memory Usage Functions:
memory_get_usage()
memory_get_peak_usage()
9. Inspect and Manage Opcache
Opcache stores precompiled script bytecode in memory. Monitor and configure it for optimal performance.
Opcache Configuration:
opcache.enable=1
opcache.memory_consumption=128
opcache.interned_strings_buffer=8
opcache.max_accelerated_files=4000
opcache.revalidate_freq=60
Inspect Opcache Status:
print_r(opcache_get_status());
10. Investigate External Dependencies
Issues might originate from databases, file systems, or external APIs. Use tools like curl
for API diagnostics and SQL query analyzers for database performance.
11. Version Compatibility
Ensure your code and libraries are compatible with the PHP version in use. Check for deprecated functions and backward-incompatible changes.
12. Automated Testing
Implement unit testing with PHPUnit to catch issues early. Use CI/CD pipelines to run tests automatically.
13. Analyze and Fix Security Issues
Use tools like PHPStan, Psalm, or PHP_CodeSniffer to detect security vulnerabilities and coding standards issues.
14. Code Review and Static Analysis
Regular code reviews and static analysis help identify potential issues before they manifest in production.
Static Analysis Tools:
- PHPStan
- Psalm
- Phan
Conclusion
Advanced PHP troubleshooting requires a systematic approach using various tools and techniques. By enabling detailed error reporting, using debuggers, monitoring performance, and leveraging automated testing and static analysis, you can efficiently identify and resolve complex PHP issues.