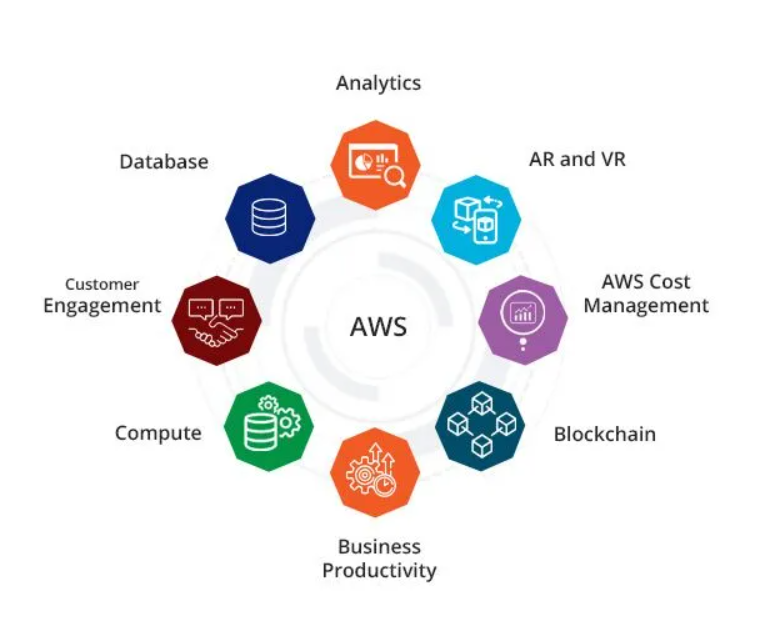
Amazon Web Services (AWS) is the world’s leading cloud computing platform that offers a wide range of cloud-based services, including computing power, storage, networking, databases, machine learning, and security. AWS enables businesses, startups, and enterprises to build scalable, cost-effective, and secure applications without having to invest in on-premises infrastructure. With over 200 fully featured services across data centers globally, AWS is used by millions of organizations to enhance operational efficiency and drive innovation.
What is AWS?
AWS is a comprehensive cloud computing platform developed by Amazon that provides Infrastructure-as-a-Service (IaaS), Platform-as-a-Service (PaaS), and Software-as-a-Service (SaaS) solutions. AWS offers a pay-as-you-go pricing model, allowing organizations to only pay for the resources they use. It supports businesses across various industries, including healthcare, finance, education, gaming, and artificial intelligence.
Key Characteristics of AWS:
- Highly Scalable: Offers automatic scaling for workloads and applications.
- Secure & Compliant: Provides enterprise-level security with compliance certifications.
- Cost-Effective: Reduces IT costs by offering flexible pricing options.
- Global Infrastructure: Spans multiple availability zones and regions worldwide.
- Innovative Technologies: Supports AI, IoT, blockchain, and analytics.
Top 10 Use Cases of AWS
- Website Hosting & Content Delivery
- AWS enables businesses to host static and dynamic websites with services like Amazon S3, Amazon EC2, and AWS CloudFront.
- Big Data Analytics
- AWS services such as Amazon Redshift, AWS Glue, and AWS Athena help businesses process and analyze large datasets efficiently.
- Machine Learning & AI
- AWS provides pre-trained AI models and machine learning frameworks through services like Amazon SageMaker, AWS DeepLens, and AWS Lex.
- Internet of Things (IoT)
- AWS IoT Core and AWS Greengrass allow organizations to securely connect and manage IoT devices at scale.
- Cloud Storage & Backup Solutions
- Amazon S3, AWS Glacier, and AWS Backup provide reliable storage and backup solutions with high availability.
- DevOps & Continuous Integration/Continuous Deployment (CI/CD)
- AWS CodePipeline, AWS CodeBuild, and AWS Lambda facilitate CI/CD pipelines for faster application development and deployment.
- Enterprise Applications & ERP Solutions
- Businesses use AWS to host ERP software like SAP and Oracle, reducing costs and increasing efficiency.
- Gaming & Media Streaming
- AWS services like Amazon GameLift and AWS Elemental enable seamless online gaming and video streaming experiences.
- Disaster Recovery & Business Continuity
- AWS ensures data redundancy and business continuity through multi-region backup and recovery solutions.
- Blockchain & Cryptocurrency
- AWS supports blockchain solutions for secure transactions using Amazon Managed Blockchain and AWS Quantum Ledger Database.
Features of AWS
- Elastic Compute Cloud (EC2) – Scalable virtual servers for hosting applications and workloads.
- Simple Storage Service (S3) – Secure and scalable object storage for backup, archive, and data sharing.
- AWS Lambda – Serverless computing for running applications without managing infrastructure.
- AWS CloudFormation – Automates infrastructure provisioning using templates.
- Amazon RDS (Relational Database Service) – Fully managed databases like MySQL, PostgreSQL, and Oracle.
- AWS Identity and Access Management (IAM) – Controls access permissions for AWS services and resources.
- AWS Auto Scaling – Automatically scales applications to handle varying traffic loads.
- Amazon DynamoDB – NoSQL database for high-performance applications.
- AWS Virtual Private Cloud (VPC) – Secure cloud networking and private IP address management.
- Amazon CloudWatch – Monitoring and logging service for AWS applications and infrastructure.
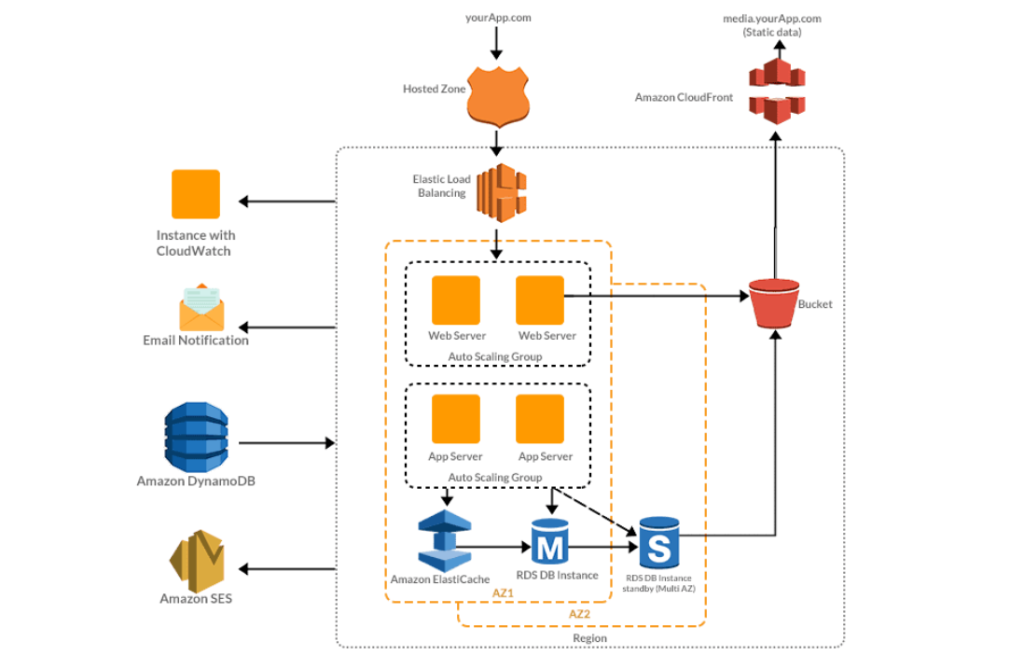
How AWS Works and Architecture
1. AWS Global Infrastructure
- AWS operates in multiple regions, availability zones (AZs), and edge locations worldwide.
- Each region consists of multiple AZs to ensure fault tolerance and disaster recovery.
2. Compute Services
- AWS EC2 instances provide virtual machines for running applications.
- AWS Lambda offers serverless computing to run code without managing servers.
3. Storage Services
- AWS S3 provides scalable object storage.
- Amazon EBS (Elastic Block Store) is used for persistent storage attached to EC2 instances.
4. Networking & Content Delivery
- AWS VPC allows users to create private cloud networks.
- AWS CloudFront delivers content with low latency using a global CDN.
5. Security & Compliance
- AWS IAM ensures secure access control.
- AWS Shield provides protection against DDoS attacks.
How to Install AWS
It seems like you’re asking how to install AWS CLI (Amazon Web Services Command Line Interface) or use AWS resources programmatically via code, but the phrase “AWS in coe” isn’t entirely clear. I’ll assume you’re referring to installing and configuring the AWS CLI or interacting with AWS services using programming code (such as Python, Terraform, etc.).
1. Installing AWS CLI
The AWS CLI (Command Line Interface) is a tool that allows you to interact with AWS services from your terminal. Here’s how to install AWS CLI:
Step 1: Install AWS CLI (Version 2)
For Windows:
- Download the AWS CLI installer for Windows from AWS CLI download page.
- Run the installer and follow the prompts.
For macOS:
You can install AWS CLI using Homebrew:
brew install awscli
Alternatively, use the official installer:
curl "https://awscli.amazonaws.com/awscli-exe-macos-x86_64.zip" -o "awscliv2.zip"
unzip awscliv2.zip
sudo ./aws/install
For Linux (Ubuntu/Debian-based):
To install AWS CLI on Linux, run:
# Download and install AWS CLI v2
curl "https://awscli.amazonaws.com/awscli-exe-linux-x86_64.zip" -o "awscliv2.zip"
unzip awscliv2.zip
sudo ./aws/install
Verify Installation:
After installation, verify that AWS CLI is installed properly by running:
aws --version
You should see an output similar to:
aws-cli/2.x.x Python/3.x.x Linux/4.x.x
2. Configure AWS CLI
Once installed, you need to configure the AWS CLI with your AWS credentials (Access Key and Secret Key).
aws configure
You’ll be prompted to enter the following:
- AWS Access Key ID: You can find this in your AWS Console under IAM (Identity and Access Management).
- AWS Secret Access Key: This will also be available in the IAM section.
- Default Region Name: This is the region you typically use, e.g.,
us-west-2
. - Default Output Format: Usually set to
json
, but you can choosetext
ortable
.
3. Install AWS SDK (For Programming Code)
If you’re interacting with AWS services programmatically, you can use AWS SDKs. Here’s how to use Python (boto3) as an example.
Step 1: Install boto3 (AWS SDK for Python)
You can install boto3, the AWS SDK for Python, using pip:
pip install boto3
Step 2: Example Python Code to Interact with AWS
Once boto3
is installed, you can write Python code to interact with AWS services.
Here’s an example Python script that lists all EC2 instances in your AWS account:
import boto3
# Create a session using your AWS credentials
ec2 = boto3.client('ec2')
# Describe EC2 instances
response = ec2.describe_instances()
# Print instance details
for reservation in response['Reservations']:
for instance in reservation['Instances']:
print(f"ID: {instance['InstanceId']}, Type: {instance['InstanceType']}, State: {instance['State']['Name']}")
Step 3: Verify Authentication
Before using the SDK, ensure you’re authenticated using AWS CLI with the aws configure
command or by setting up your credentials file.
Alternatively, you can provide your AWS Access Key ID and Secret Access Key programmatically using:
import boto3
# Use AWS access keys directly (if not using configured profile)
ec2 = boto3.client('ec2', aws_access_key_id='your-access-key',
aws_secret_access_key='your-secret-key', region_name='us-west-2')
However, using IAM roles and AWS CLI configuration is the recommended and safer approach.
4. Automate AWS Infrastructure with Terraform
You can use Terraform to provision and manage AWS resources. Here’s an example of provisioning an EC2 instance with Terraform:
Step 1: Install Terraform
Download and install Terraform from the official site.
For Linux (Ubuntu):
sudo apt-get update
sudo apt-get install terraform
For macOS:
brew install terraform
Step 2: Configure Terraform to Use AWS
Create a main.tf
file to configure an AWS provider and resource.
# Configure AWS provider
provider "aws" {
region = "us-west-2"
}
# Provision an EC2 instance
resource "aws_instance" "example" {
ami = "ami-0c55b159cbfafe1f0" # Use your preferred AMI ID
instance_type = "t2.micro"
tags = {
Name = "MyInstance"
}
}
Step 3: Apply Terraform Configuration
Initialize and apply the Terraform configuration:
terraform init
terraform apply
This will provision the EC2 instance on AWS based on the configuration.
5. Monitor and Manage AWS with CloudWatch and CloudTrail
You can use CloudWatch to monitor AWS services and CloudTrail to log API activity.
For example, using AWS CLI to create a CloudWatch alarm:
aws cloudwatch put-metric-alarm --alarm-name "HighCPUAlarm" \
--metric-name "CPUUtilization" --namespace "AWS/EC2" \
--statistic "Average" --period 300 --threshold 80 \
--comparison-operator "GreaterThanThreshold" \
--dimensions "Name=InstanceId,Value=i-12345678" \
--evaluation-periods 2 --alarm-actions arn:aws:sns:us-west-2:123456789012:MyTopic
This creates an alarm that triggers an SNS notification if CPU utilization exceeds 80%.
Basic Tutorials of AWS: Getting Started
Step 1: Create an EC2 Instance
- Log in to the AWS Management Console.
- Navigate to EC2 > Launch Instance.
- Select an Amazon Machine Image (AMI) (e.g., Ubuntu, Windows Server).
- Choose an Instance Type (e.g., t2.micro for free tier).
- Configure security groups and launch the instance.
Step 2: Create an S3 Bucket
- Go to S3 Service in AWS.
- Click Create Bucket, set a unique bucket name, and choose a region.
- Configure permissions and upload files.
Step 3: Deploy a Serverless Function with AWS Lambda
- Open AWS Lambda from the AWS Console.
- Click Create Function and select Author from Scratch.
- Choose a runtime (e.g., Python, Node.js).
- Upload your function code and deploy.
Step 4: Set Up a CloudWatch Monitoring Dashboard
- Go to Amazon CloudWatch.
- Click Create Dashboard.
- Add widgets for CPU Usage, Memory Utilization, and Network Metrics.