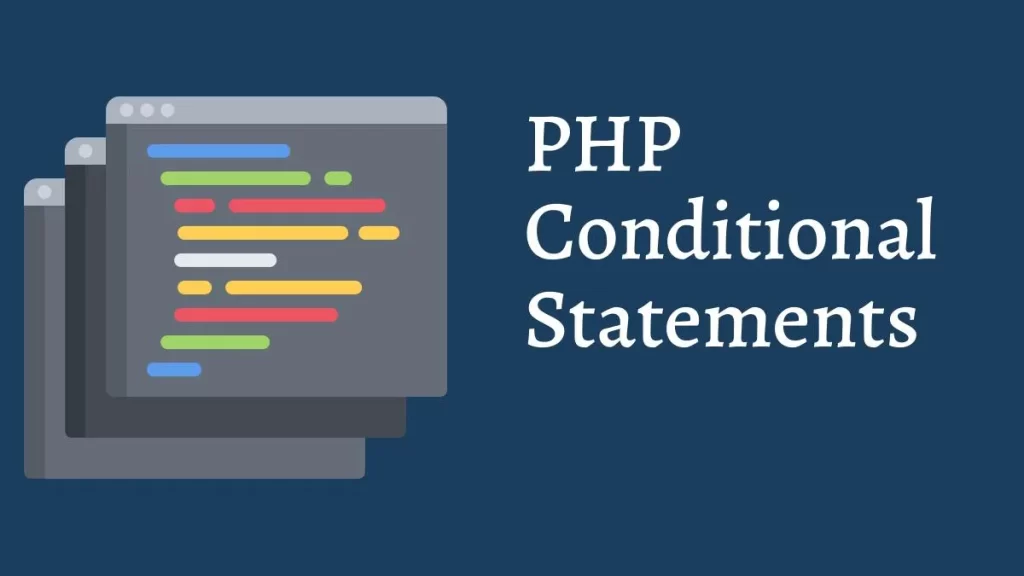
In PHP, conditional statements are used to perform different actions based on different conditions.
The most commonly used conditional statements in PHP are:
- if Statement.
- if-else Statement
- If-elseif-else Statement
- Switch statement
1. if Statement : If statement is used to test a condition and execute a block of code if the condition is true.
Syntax:
if(condition)
{
//code to be executed
}
Flowchart:
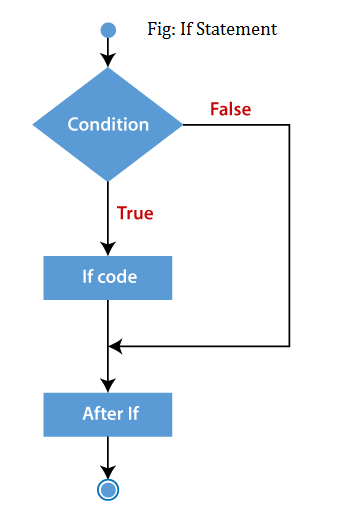
Example:
$num = 20;
if ($num > 5)
{
echo "The number is greater than 5.";
}
2. if-else Statement: This statement is used to check a condition and execute one block of code if the condition is true and another block of code if the condition is false.
syntax:
if(condition)
{
//code to be executed if true
}
else
{
//code to be executed if false
}
Flowchart:
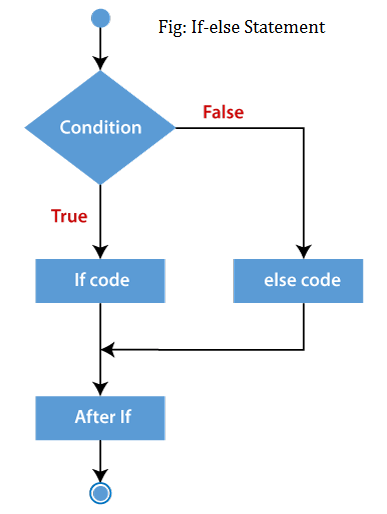
Example:
$num = 5;
if ($num > 10)
{
echo "The number is greater than 10.";
}
else
{
echo "The number is less than or equal to 5.";
}
3. If-else-if-else: This statement is used to Check multiple conditions and execute different blocks of code based on those conditions.
Syntax:
if (condition1)
{
//code to be executed if condition1 is true
} elseif (condition2)
{
//code to be executed if condition2 is true
} elseif (condition3)
{
//code to be executed if condition3 is true
...
} else
{
//code to be executed if all given conditions are false
}
Flowchart:
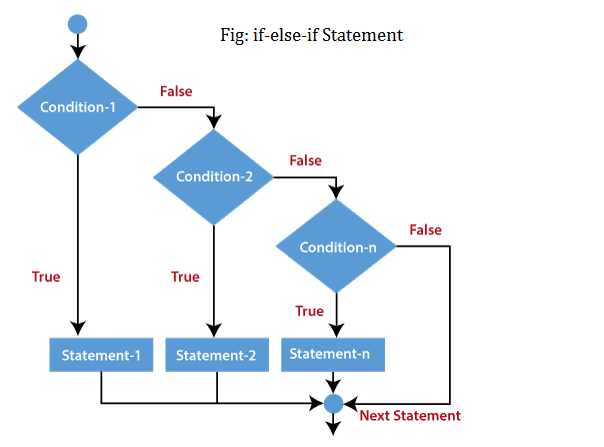
Example:
$num = 7;
if ($num > 10)
{
echo "The number is greater than 10.";
}
else if ($num > 5)
{
echo "The number is greater than 5 but less than or equal to 10.";
}
else
{
echo "The number is less than or equal to 5.";
}
4. Switch Statement:
PHP switch statement is used to execute one statement from multiple conditions. It works like PHP if-else-if statement.
Syntax:
switch(expression)
{
case value1:
//code to be executed
break;
case value2:
//code to be executed
break;
......
default:
code to be executed if all cases are not matched;
}
Example:
<?php$i = "2";switch ($i) { case 0: echo "i equals 0"; break; case 1: echo "i equals 1"; break; case 2: echo "i equals 2"; break; default: echo "i is not equal to 0, 1 or 2";}?>