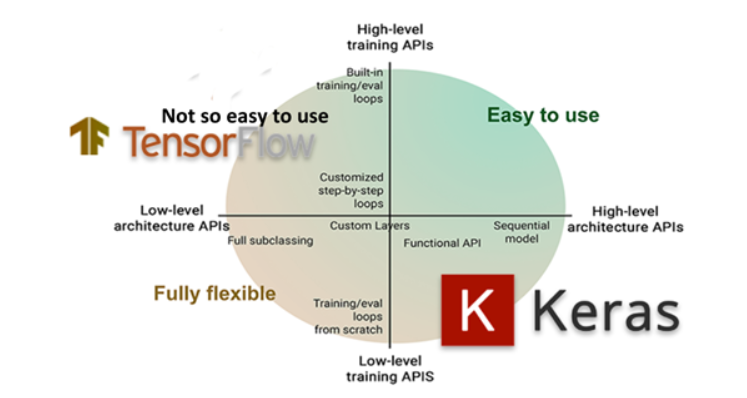
Keras is a high-level deep learning framework that provides an easy-to-use interface for building, training, and deploying deep learning models. It is written in Python and can run on top of popular deep learning backends like TensorFlow, Theano, and Microsoft Cognitive Toolkit (CNTK). Keras is designed with user-friendliness, modularity, and extensibility in mind, making it a go-to tool for researchers, engineers, and developers exploring artificial intelligence and machine learning.
What is Keras?
Keras simplifies building deep learning models by abstracting the complexities of backend computations. It allows users to focus more on designing and iterating models rather than dealing with the low-level details of tensor operations. Some of its key characteristics include:
- User-Friendly: Offers a clean and intuitive API for fast prototyping.
- Extensibility: Supports custom layers, metrics, and loss functions.
- Cross-Platform Compatibility: Runs seamlessly on CPUs, GPUs, and TPUs.
- Wide Adoption: Used in academic research, industrial applications, and startups worldwide.
Top 10 Use Cases of Keras
- Image Classification: Keras is widely used for training models that classify images into categories, such as identifying objects in pictures or detecting facial expressions.
- Natural Language Processing (NLP): Used for tasks like text classification, sentiment analysis, and language translation.
- Speech Recognition: Enables the development of speech-to-text systems and voice assistants.
- Recommendation Systems: Powers personalized recommendations, such as those used by e-commerce and streaming platforms.
- Healthcare Applications: Assists in medical imaging diagnostics, such as detecting anomalies in X-rays or MRIs.
- Anomaly Detection: Detects fraudulent activities, such as credit card fraud, and irregular patterns in financial transactions.
- Time Series Analysis: Used for forecasting trends, stock prices, and weather patterns.
- Autonomous Driving: Facilitates perception and decision-making systems for self-driving cars.
- Generative Models: Enables the creation of realistic images, videos, and music using GANs (Generative Adversarial Networks).
- Robotics: Helps robots learn motor skills, object recognition, and navigation.
What are the Features of Keras?
- Modularity: Components like layers, loss functions, and optimizers are fully modular and easily configurable.
- Pretrained Models: Provides access to a library of pretrained models such as VGG, ResNet, and Inception for transfer learning.
- Multiple Backend Support: Works with TensorFlow, Theano, or CNTK for flexibility in deployment.
- Extensive Documentation: Offers detailed guides and examples for beginners and advanced users.
- Customizability: Supports building custom neural network architectures and layers.
- Built-in Support for GPUs: Accelerates model training by utilizing GPU hardware.
- Integration with Other Libraries: Compatible with NumPy, Pandas, and Matplotlib for preprocessing and visualization.
- Easy Debugging: Errors are reported with clear and helpful messages, simplifying the debugging process.
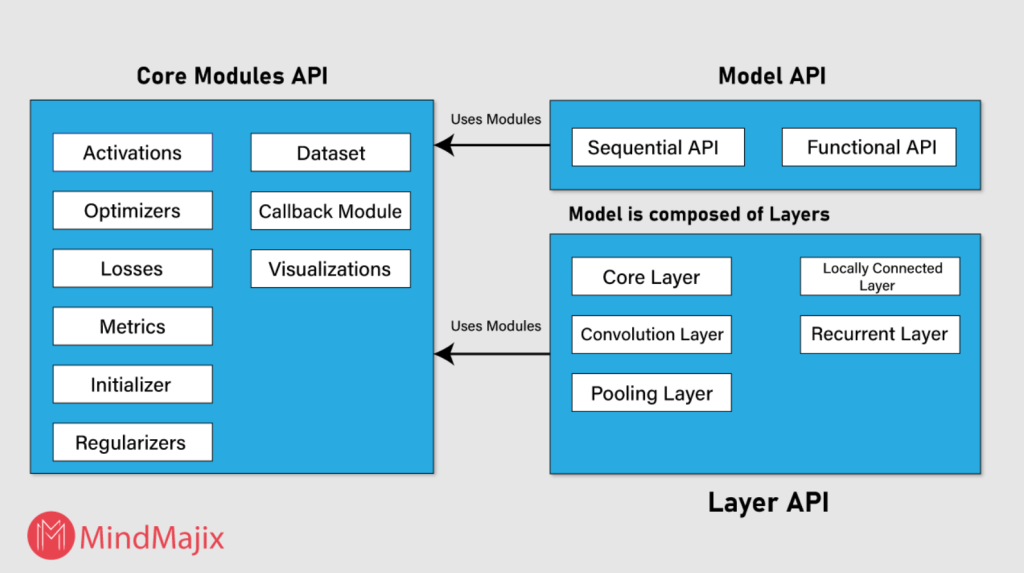
How Keras Works and Its Architecture
Keras provides a high-level abstraction for building neural networks. Its architecture consists of the following key components:
- Models:
- Sequential API: Allows building models layer-by-layer.
- Functional API: Facilitates creating complex models with multiple inputs and outputs.
- Layers: Layers are the building blocks of Keras models and can be stacked to create a neural network.
- Backend Engine: Keras uses a backend engine (e.g., TensorFlow) to perform numerical computations.
- Loss Functions: Specifies the objective that the model should optimize.
- Optimizers: Algorithms like SGD, Adam, and RMSProp adjust model weights to minimize the loss function.
- Metrics: Evaluate the model’s performance during training and testing.
How to Install Keras
To install Keras, follow these steps:
1.Install a Backend Framework: Keras requires a backend engine. You can install TensorFlow, JAX, or PyTorch. For example, to install TensorFlow, use:
pip install tensorflow
2.Install Keras: Once the backend is installed, install Keras using pip:
pip install keras
3. Verify Installation: To confirm successful installation, run the following in Python:
import keras
print(keras.__version__)
This should display the installed Keras version without errors.
Basic Tutorials of Keras: Getting Started
To get started with Keras, consider the following steps:
1.Import Necessary Libraries:
import keras
from keras.models import Sequential
from keras.layers import Dense
2.Load and Preprocess Data: Keras provides utilities to load datasets like MNIST. Preprocess the data by normalizing and reshaping as required.
3. Build the Model:
model = Sequential()
model.add(Dense(128, activation='relu', input_shape=(input_dim,)))
model.add(Dense(10, activation='softmax'))
4. Compile the Model: Specify the optimizer, loss function, and metrics:
model.compile(optimizer='adam', loss='sparse